While cycle loop in Java example
While cycle Java basic example with array of strings.
MainClass.java
MainClass.java
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "Hello", "people", "hello", "world!" };
int i = 0;
while ( i < arrayOfString.length ){
System.out.println(arrayOfString[i]);
i++;
}
}
}
396LW NO topic_id
AD
Další témata ....(Topics)
Try two way in Eclipse IDE:
1.) Import the library project into your Eclipse workspace.
Click File > Import,
select Android > Existing Android Code into Workspace, and browse to the copy of the library project to import it.
If project not visible with checkbox try next step as see below.
2.) Click
File > New > Other
select Android > Existing Android Code into Workspace, and browse to the copy of the library project to import it.
1.) Import the library project into your Eclipse workspace.
Click File > Import,
select Android > Existing Android Code into Workspace, and browse to the copy of the library project to import it.
If project not visible with checkbox try next step as see below.
2.) Click
File > New > Other
select Android > Existing Android Code into Workspace, and browse to the copy of the library project to import it.
Brand | Motorola |
Model (codename) | Droid Razr |
Price (cena, včetně DPH v KCZ) | 10800 / 06.2012 |
Display size in Inch (v palcích) | 4.3 |
Display-resolution | 540x960 |
Dotek-typ | capacitive |
CPU typ | TI 4430 |
CPU MHz | 1.2 GB |
CPU core | 2 |
L2 cache | |
RAM | 1024 |
ROM | 15600 |
GPU | SGX540 |
NenaMark2 Benchmark | |
GPU-GLBenchmark | 3299 |
Baterie mAh | 1780 |
Foto MPx | 8 |
Autofocus | AF |
Video | HD video 30 frames/s |
Official Android ICS | Google Android 2.3.5 (Gingerbread) |
CyanogenMod support | |
Dotek-prstů-max | 10 |
Display-ppi | 256 |
Display-retina | 79% |
Networks | |
Connectivity | GSM: 850/900/1800/1900 MHz, EDGE, GPRS 3G: 900/2100 MHz, HSDPA, HSUPA, HSPA+ Bluetooth: 4.0 (EDR, A2DP, FTP, PBAP, AVRCP) Wi-Fi: 802.11b/g/n PC: microUSB, USB 2.0, microHDMI Senzors: proximity, gyroskop, akcelerometr GPS: yes, A-GPS, digital compas |
Note | Super AMOLED Display |
Motorola Droid Razr image
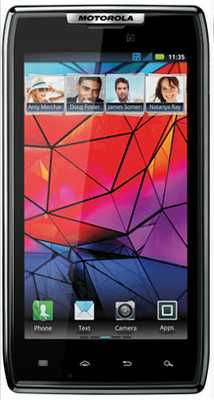
Warning: The application may be doing too much work on its main thread
Try this sorce code:
Try this sorce code:
import android.os.StrictMode;
public class MyActivity extends Activity {
static{
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
@Override
public void onCreate(Bundle savedInstanceState) {
//.................. etc.
HTC Sensation 4G cena od 11 000 KCZ Kč (únor.2012)
Spokojenost uživatelů nadprůměrná.
HTC Sensation 4G je chytrý telefon s operačním systémem Android.
HTC Sensation 4G je (22.února2012) 10. nejpoužívanějším chytrým telefonem u programu Sky Map viz tabulka.
Výrobce HTC
Kompatibilní sítě GSM / GPRS / EDGE
850 900 1800 1900 MHz
UMTS / HSPA
850 1900 2100 nebo 900 1700 2100 MHz
HSDPA 14,4 Mbps
HSUPA 5.76 Mbps
První předvedení 19.května 2011
Zaváděcí cena US 199,99 dolarů
Nástupce HTC Sensation XE, HTC 4G Amaze
Rozměry 126.1 mm (4.96 v) H
65.4 mm 2,57 in) W
11.3 mm (0,44 in) D
Hmotnost 148 g
Operační systém Android 2.3.3 Gingerbread (upgrade až na 2.3.4),
HTC Sense 3.0 overlay
Procesor:
Dual-core CPU, 1.2 GHz Qualcomm MSM8260 Snapdragon, Sensation XE dual-core 1,5 GHz Qualcomm MSM8260 Snapdragon, Sensation XL jednoho jádra 1,5 GHz Qualcomm MSM8255 Snapdragon
GPU Qualcomm adreno 220, Sensation XL adreno 205
Paměť 768 MB RAM
4 GB (1 GB uživatelsky dostupných)
Paměť vyjmutelná: 8 GB microSD 2.0, podporuje až 32 GB
Baterie 1520 mAh vnitřní dobíjecí Li-ion vyměnitelné
Datové vstupy A-GPS, čidlo okolního osvětlení, digitální kompas, G-senzor, gyroskop, multi-touch kapacitní dotykový displej, snímač
Displej 4.3 v (110 mm) kapacitní S-LCD dotykový displej Gorilla skla s QHD (540 × 960) rozlišením na 256.15 PPI
Fotoaparát na zadní straně s 8 miliony pixelů (3264 x 2448) s automatickým ostřením a dvojitým LED bleskem a 1080p HD video záznamu @ 30 snímků / s, kohoutek k zaměření s digitálním zoomem
Fotoaparát na přední straně VGA pevné zaostření barevná kamera (0,3 megapixelů)
Kompatibilní média formáty audio AAC, AMR, OGG, M4A, MIDI, MP3, WAV, WMA
Video 1920 × 1080 (1080p HD) @ 30 snímků / s - 3GP, .3 G2, MP4, WMV, AVI, XVID
Vyzváněcí tóny a oznámení všechny kompatibilní audio formáty
Vibrace
Připojení 3.5 mm TRRS konektor, Bluetooth 3.0 s A2DP, FM přijímač (87,5 - 108 MHz) s RDS, Micro USB 2.0 (5-pin) port s Mobile High-Definition Link (MHL) pro USB nebo HDMI připojení, Wi-Fi 802.11b/g/n
Zdroj: wikipedia
HTC Sensation 4G photo pic image
Zdroj obrázku: wikipedia
Spokojenost uživatelů nadprůměrná.
HTC Sensation 4G je chytrý telefon s operačním systémem Android.
HTC Sensation 4G je (22.února2012) 10. nejpoužívanějším chytrým telefonem u programu Sky Map viz tabulka.
Výrobce HTC
Kompatibilní sítě GSM / GPRS / EDGE
850 900 1800 1900 MHz
UMTS / HSPA
850 1900 2100 nebo 900 1700 2100 MHz
HSDPA 14,4 Mbps
HSUPA 5.76 Mbps
První předvedení 19.května 2011
Zaváděcí cena US 199,99 dolarů
Nástupce HTC Sensation XE, HTC 4G Amaze
Rozměry 126.1 mm (4.96 v) H
65.4 mm 2,57 in) W
11.3 mm (0,44 in) D
Hmotnost 148 g
Operační systém Android 2.3.3 Gingerbread (upgrade až na 2.3.4),
HTC Sense 3.0 overlay
Procesor:
Dual-core CPU, 1.2 GHz Qualcomm MSM8260 Snapdragon, Sensation XE dual-core 1,5 GHz Qualcomm MSM8260 Snapdragon, Sensation XL jednoho jádra 1,5 GHz Qualcomm MSM8255 Snapdragon
GPU Qualcomm adreno 220, Sensation XL adreno 205
Paměť 768 MB RAM
4 GB (1 GB uživatelsky dostupných)
Paměť vyjmutelná: 8 GB microSD 2.0, podporuje až 32 GB
Baterie 1520 mAh vnitřní dobíjecí Li-ion vyměnitelné
Datové vstupy A-GPS, čidlo okolního osvětlení, digitální kompas, G-senzor, gyroskop, multi-touch kapacitní dotykový displej, snímač
Displej 4.3 v (110 mm) kapacitní S-LCD dotykový displej Gorilla skla s QHD (540 × 960) rozlišením na 256.15 PPI
Fotoaparát na zadní straně s 8 miliony pixelů (3264 x 2448) s automatickým ostřením a dvojitým LED bleskem a 1080p HD video záznamu @ 30 snímků / s, kohoutek k zaměření s digitálním zoomem
Fotoaparát na přední straně VGA pevné zaostření barevná kamera (0,3 megapixelů)
Kompatibilní média formáty audio AAC, AMR, OGG, M4A, MIDI, MP3, WAV, WMA
Video 1920 × 1080 (1080p HD) @ 30 snímků / s - 3GP, .3 G2, MP4, WMV, AVI, XVID
Vyzváněcí tóny a oznámení všechny kompatibilní audio formáty
Vibrace
Připojení 3.5 mm TRRS konektor, Bluetooth 3.0 s A2DP, FM přijímač (87,5 - 108 MHz) s RDS, Micro USB 2.0 (5-pin) port s Mobile High-Definition Link (MHL) pro USB nebo HDMI připojení, Wi-Fi 802.11b/g/n
Zdroj: wikipedia
HTC Sensation 4G photo pic image
Zdroj obrázku: wikipedia
Insert into your default start up activity tag inten-filter tag with action MAIN and category LAUNCHER
AndroidManifest.xml
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="//schemas.android.com/apk/res/android"
package="com.example.blabol"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="19" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Editace: 2011-10-04 08:57:30
Počet článků v kategorii: 396
Url:while-cycle-loop-in-java-example