Preferences settings save open read write application Android example
Android app setup preferences, settings, open, read, write, onStop(), onPause(), getSharedPreferences(), SharedPreferences.Editor getInt(), getBoolean(), getString() , putInt(), putString(), putBoolean() example source code.
import android.content.SharedPreferences;
public class MainClass extends Activity {
public static final String PREFERENCES_NAME = "MyPrefsFile";
String myNewString;
int myNewInt;
Boolean myNewBool;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// read old settings if exist
SharedPreferences settings = getSharedPreferences(PREFERENCES_NAME, 0);
String sDefault = "Hello!";
String sStringFromPrefFile = settings.getString("myString", sDefault); // new text or default Hello!
int nDefaultIndex = 2; //
int nIndexFromPrefFile = settings.getInt("myInt", nDefaultIndex); // 4 or default 2
Boolean bDefault = false;
Boolean bFromPrefFile = settings.getBoolean("silentMode", bDefault); // true or default false
// new settings will saved in onStop or onPause
myNewString = "new text";
myNewInt = 4;
myNewBool = true;
}
@Override
protected void onPause() {
super.onPause();
Toast.makeText(getApplicationContext(), "onPause "
, Toast.LENGTH_SHORT).show();
savePreferences();
}
@Override
protected void onStop() {
super.onStop();
savePreferences();
}
/**save settings*/
public void savePreferences(){
try {
SharedPreferences settings = getSharedPreferences(PREFERENCES_NAME, 0);
SharedPreferences.Editor editor = settings.edit();
editor.putString("myString", myNewString);
editor.putInt("nMyInt", myNewInt);
editor.putBoolean("silentMode", myNewBool); //
editor.commit();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
396LW NO topic_id
AD
Další témata ....(Topics)
How get application version, sdk version, package name defined in the AndroidManifest file programmically Android sample.
MainClass.java onCreate()
AndroidManifes.xml
MainClass.java onCreate()
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// in onCreate
PackageInfo pinfo = this.getPackageManager().getPackageInfo(getPackageName(), 0);
String sVersionCode = pinfo.versionCode; // 1
String sVersionName = pinfo.versionName; // 1.0
String sPackName = getPackageName(); // cz.okhelp.my_app
int nSdkVersion = Integer.parseInt(Build.VERSION.SDK); // 7
int nSdkVers = Build.VERSION.SDK_INT; // 7
}
AndroidManifes.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="//schemas.android.com/apk/res/android"
package="cz.okhelp.my_app"
android:versionCode="1"
android:versionName="1.0">
<uses-sdk android:minSdkVersion="7" />
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".Add_view_to_tableActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Terms Screen size, density, density independent pixel, resolution as a picture - pictogram.
Test your knowledge
Q: How to find out the phone screen size?
A: (By length of display diagonale in inch - Not to measure a diagonal of device!!!)
Q: What resolution has 720 x 1280 display?
A: (921600 pixels)
Q: What does it mean "240 dpi" screen density?
A: (Display have density 240 x 240 dots - "Tri-color LED etc." - per every physical (real) square inch. If you have icon 240x240 pixels, this will just occupy an area of one square inch on the display.)
Q: Phone have screen density 240 dpi. Image for 160 dpi screen density have size 128x 128 pixels. What will be the size of the image for 240 dpi screen density?
A: (Calculate the virtual pixels size. 128 * (240/160) = 192. You have to resize image to new size 192 x 192 physical pixels and put into folder drawable-hdpi (high) ~240dpi for phone with screen density 240 dpi. ) or use density independend pixels 128dp x 128dp.
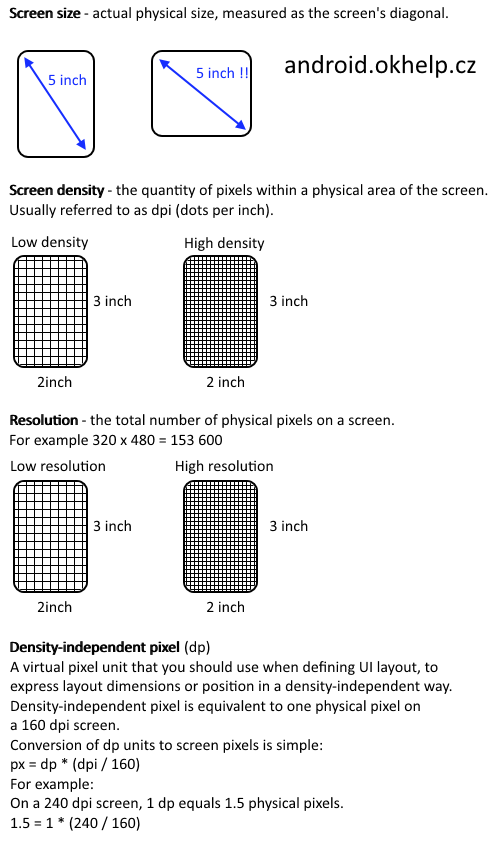
Test your knowledge
Q: How to find out the phone screen size?
A: (By length of display diagonale in inch - Not to measure a diagonal of device!!!)
Q: What resolution has 720 x 1280 display?
A: (921600 pixels)
Q: What does it mean "240 dpi" screen density?
A: (Display have density 240 x 240 dots - "Tri-color LED etc." - per every physical (real) square inch. If you have icon 240x240 pixels, this will just occupy an area of one square inch on the display.)
Q: Phone have screen density 240 dpi. Image for 160 dpi screen density have size 128x 128 pixels. What will be the size of the image for 240 dpi screen density?
A: (Calculate the virtual pixels size. 128 * (240/160) = 192. You have to resize image to new size 192 x 192 physical pixels and put into folder drawable-hdpi (high) ~240dpi for phone with screen density 240 dpi. ) or use density independend pixels 128dp x 128dp.
Samsung Galaxy S II cena od 10 000 KCZ Kč (únor.2012).
Asi nejlepší smartphone (cena : vlastnosti) telefon s Androidem roku 2011.
Samsung Galaxy S II (GT-I9100) je chytrý telefon běžící na operačním systému Android, který byl představen firmou Samsung 13. února 2011.
Samsung Galaxy S II je (22.února2012) nejpoužívanějším chytrým telefonem u programu Sky Map viz tabulka.
Proč je Samsung Galaxy S2 tak oblíben?
Galaxy S II má 1.2 GHz dual-core "Exynos" procesor, 1 GB RAM, 10,8 cm WVGA AMOLED Super Plus displej a fotoaparát s 8 miliony pixelů i s bleskem a plné vysoké rozlišení 1080p nahrávání videa. Je to jedno z prvních zařízení, které nabízí mobilní high-definition Link (MHL), který umožňuje až 1080p nekomprimovaného obrazového výstupu HDMI. USB On-The-Go (USB OTG) je podporováno.
Vyměnitelná baterie na Galaxy S II vydrží až deset hodin při intenzivním používání, nebo dva dny lehčí provoz. Podle společnosti Samsung, Galaxy S II je schopen poskytovat 9 hodin hovoru v 3G a 18,3 hodin na 2G síti.
Samsung Galaxy S2 photo pic image
Zdroj obrázku: wikipedia
Asi nejlepší smartphone (cena : vlastnosti) telefon s Androidem roku 2011.
Samsung Galaxy S II (GT-I9100) je chytrý telefon běžící na operačním systému Android, který byl představen firmou Samsung 13. února 2011.
Samsung Galaxy S II je (22.února2012) nejpoužívanějším chytrým telefonem u programu Sky Map viz tabulka.
Proč je Samsung Galaxy S2 tak oblíben?
Galaxy S II má 1.2 GHz dual-core "Exynos" procesor, 1 GB RAM, 10,8 cm WVGA AMOLED Super Plus displej a fotoaparát s 8 miliony pixelů i s bleskem a plné vysoké rozlišení 1080p nahrávání videa. Je to jedno z prvních zařízení, které nabízí mobilní high-definition Link (MHL), který umožňuje až 1080p nekomprimovaného obrazového výstupu HDMI. USB On-The-Go (USB OTG) je podporováno.
Vyměnitelná baterie na Galaxy S II vydrží až deset hodin při intenzivním používání, nebo dva dny lehčí provoz. Podle společnosti Samsung, Galaxy S II je schopen poskytovat 9 hodin hovoru v 3G a 18,3 hodin na 2G síti.
Samsung Galaxy S2 photo pic image

Zdroj obrázku: wikipedia
Not request focus EditText if startup Android - hide keyboard if startup.
Remove: from EditText
Create a LinearLayout and set the:
attributes android:focusable="true" android:focusableInTouchMode="true"
Remove:
Create a LinearLayout and set the:
attributes android:focusable="true" android:focusableInTouchMode="true"
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:focusable="true"
android:focusableInTouchMode="true"
android:orientation="vertical" >
</LinearLayout>
Date getDate is deprecated Java Android example code:
long dayInMili = 100000000;
GregorianCalendar calToDay = new GregorianCalendar();
int nD = calToDay.get((Calendar.MILLISECOND)); // +dayInMili;
System.out.println(nD);
long lTime = calToDay.getTimeInMillis();
System.out.println(lTime);
Date dtA = new Date(lTime); // today
///@SuppressWarnings("deprecation")
int nD1 = dtA.getDate(); // DEPRECATED Day Of Month 1 - 31
System.out.println(nD1+" nD1");
GregorianCalendar cal = new GregorianCalendar();
int nD2 = calToDay.get(Calendar.DATE); // Day Of Month
System.out.println(nD2+" nD2");
cal.setTimeInMillis(lTime+dayInMili);// setTime(dtA);
int nD3 = cal.get(Calendar.DATE); // Day Of Month + 1 day
System.out.println(nD3+" nD3");
Editace: 2011-09-19 15:11:38
Počet článků v kategorii: 396
Url:preferences-settings-save-open-red-application-android-example