Click Handler
Click Handler Android Java example source code. Open new class as activity and open URL in browser.
In layout.xml file:
In string.xml
In MainClass.java file
In layout.xml file:
android:layout_height="wrap_content" android:onClick="@string/clickHandler">
In string.xml
myClickHandler
In MainClass.java file
public void myClickHandler(View view) {
switch (view.getId()) {
// open new class as activity
case R.id.btnOpenClass: {
Intent pictureActivity = new Intent(getBaseContext(),
MyClass.class);
startActivity(pictureActivity);
}
break;
case R.id.btnAbout: {
//aboutDialogCreate();
}
break;
// open url
case R.id.btnHome: {
String url = "//android.okhelp.cz/";
Intent i = new Intent(Intent.ACTION_VIEW);
i.setData(Uri.parse(url));
startActivity(i);
}break;
}
}// end myClickHandler
// listener .. if click on button will scrolling to mTextView bottom
private OnClickListener mButtonListener = new OnClickListener() {
public void onClick(View v) {
// do something when the button is clicked
int nBottom = mTextView.getBottom();
hScrollView.scrollTo(0, nBottom);
}
};
396LW NO topic_id
AD
Další témata ....(Topics)
Android development example source code
Get supported language:
// import
import android.speech.tts.TextToSpeech;
import android.speech.tts.TextToSpeech.OnInitListener;
// you have to add implementation
public class Main extends Activity implements TextToSpeech.OnInitListener {
private int _langTTSavailable = -1; // set up in onInit method
// declaration
private TextToSpeech mTts;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// assigned handle - initialisation
mTts = new TextToSpeech(this,
(OnInitListener) this // TextToSpeech.OnInitListener
);
}
// Implements TextToSpeech.OnInitListener.
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
// Set preferred language to US english.
_langTTSavailable = mTts.setLanguage(Locale.US); // Locale.FRANCE etc.
if (_langTTSavailable == TextToSpeech.LANG_MISSING_DATA ||
_langTTSavailable == TextToSpeech.LANG_NOT_SUPPORTED) {
} else if ( _langTTSavailable >= 0) {
mTts.speak("Good morning",
TextToSpeech.QUEUE_FLUSH, // Drop all pending entries in the playback queue.
null);
}
} else {
// Initialization failed.
}
}
@Override
public void onDestroy() {
// TTS shutdown!
if (mTts != null) {
mTts.stop();
mTts.shutdown();
}
super.onDestroy();
}
}
Get supported language:
private TextToSpeech mTts;
// public void onInit(int status){
int result;
String s;
result = mTts.setLanguage( Locale. CANADA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CANADA not supported<br>" ;}else{s+=" CANADA supported<br>";}
result = mTts.setLanguage( Locale. CANADA_FRENCH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CANADA_FRENCH not supported<br>" ;}else{s+=" CANADA_FRENCH supported<br>";}
result = mTts.setLanguage( Locale. CHINA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CHINA not supported<br>" ;}else{s+=" CHINA supported<br>";}
result = mTts.setLanguage( Locale. CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CHINESE not supported<br>" ;}else{s+=" CHINESE supported<br>";}
result = mTts.setLanguage( Locale. ENGLISH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ENGLISH not supported<br>" ;}else{s+=" ENGLISH supported<br>";}
result = mTts.setLanguage( Locale. FRANCE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " FRANCE not supported<br>" ;}else{s+=" FRANCE supported<br>";}
result = mTts.setLanguage( Locale. FRENCH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " FRENCH not supported<br>" ;}else{s+=" FRENCH supported<br>";}
result = mTts.setLanguage( Locale. GERMAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " GERMAN not supported<br>" ;}else{s+=" GERMAN supported<br>";}
result = mTts.setLanguage( Locale. GERMANY ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " GERMANY not supported<br>" ;}else{s+=" GERMANY supported<br>";}
result = mTts.setLanguage( Locale. ITALIAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ITALIAN not supported<br>" ;}else{s+=" ITALIAN supported<br>";}
result = mTts.setLanguage( Locale. ITALY ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ITALY not supported<br>" ;}else{s+=" ITALY supported<br>";}
result = mTts.setLanguage( Locale. JAPAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " JAPAN not supported<br>" ;}else{s+=" JAPAN supported<br>";}
result = mTts.setLanguage( Locale. JAPANESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " JAPANESE not supported<br>" ;}else{s+=" JAPANESE supported<br>";}
result = mTts.setLanguage( Locale. KOREA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " KOREA not supported<br>" ;}else{s+=" KOREA supported<br>";}
result = mTts.setLanguage( Locale. KOREAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " KOREAN not supported<br>" ;}else{s+=" KOREAN supported<br>";}
result = mTts.setLanguage( Locale. PRC ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " PRC not supported<br>" ;}else{s+=" PRC supported<br>";}
result = mTts.setLanguage( Locale. ROOT ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ROOT not supported<br>" ;}else{s+=" ROOT supported<br>";}
result = mTts.setLanguage( Locale. SIMPLIFIED_CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " SIMPLIFIED_CHINESE not supported<br>" ;}else{s+=" SIMPLIFIED_CHINESE supported<br>";}
result = mTts.setLanguage( Locale. TAIWAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " TAIWAN not supported<br>" ;}else{s+=" TAIWAN supported<br>";}
result = mTts.setLanguage( Locale. TRADITIONAL_CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " TRADITIONAL_CHINESE not supported<br>" ;}else{s+=" TRADITIONAL_CHINESE supported<br>";}
result = mTts.setLanguage( Locale. UK ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " UK not supported<br>" ;}else{s+=" UK supported<br>";}
result = mTts.setLanguage( Locale. US ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " US not supported<br>" ;}else{s+=" US supported<br>";}
Eclipse make own color of toolbars, windows, status bar etc.
https://github.com/jeeeyul/eclipse-themes/wiki/Alternative-Install
https://github.com/jeeeyul/eclipse-themes/wiki/Alternative-Install
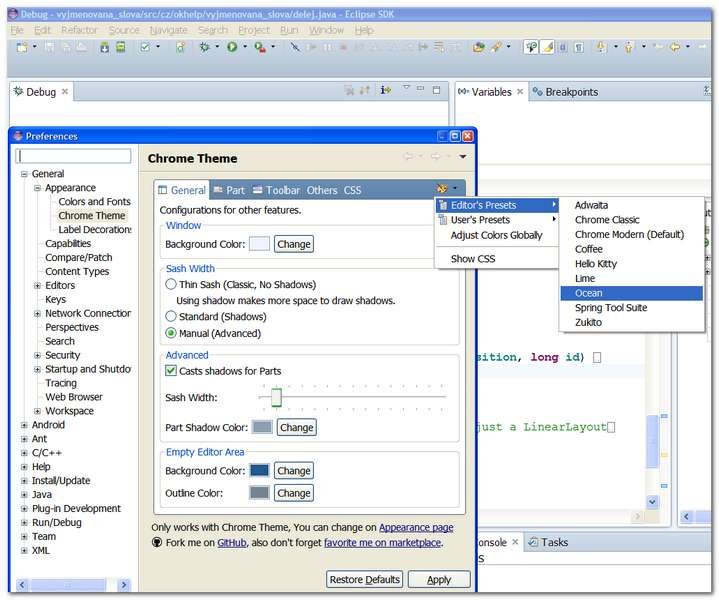
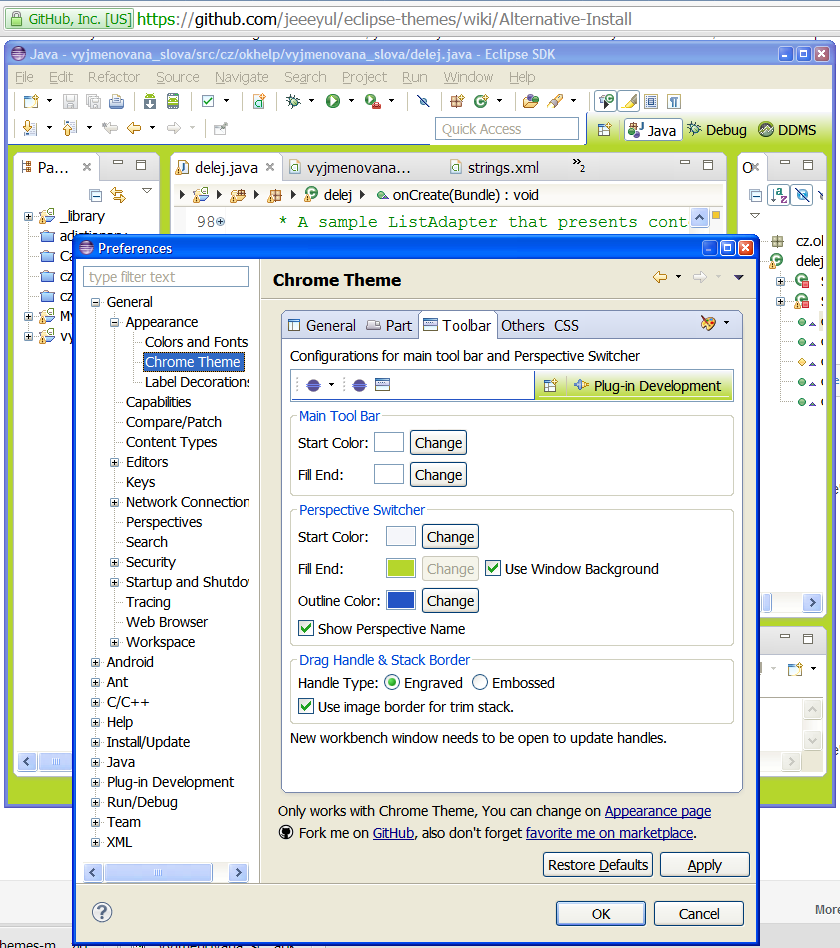
Hide module - open dialog: File > Project Structure Ctrl + Alt + Shift + S
Hide module: in opened Dialog select module which will hidden and click on minus (left upper corner)
If module is hidden, you can permanetly delete module from disk. But if you want using module in future, copy module into other folder (not into AndroidProjects folder and his subbfolders) and delete module permanetly from project and disc. Right mouse click on module and select from menu Delete.
If you want import the backup copy to project, use: File>New>Import mudule
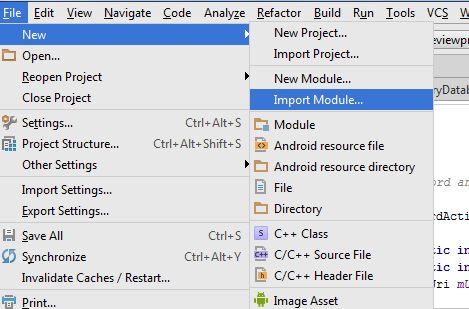

Hide module: in opened Dialog select module which will hidden and click on minus (left upper corner)
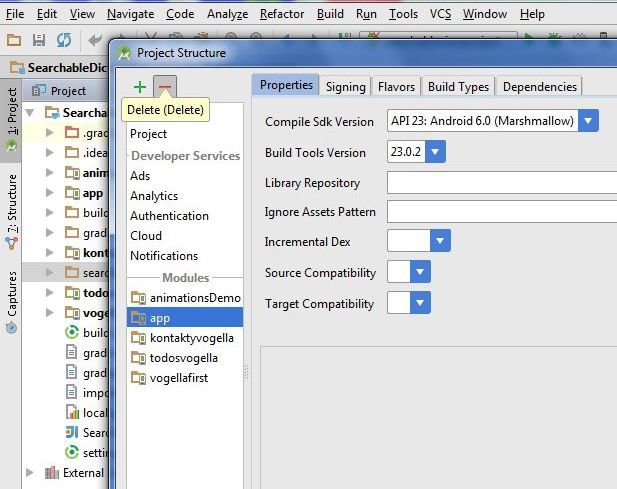
If module is hidden, you can permanetly delete module from disk. But if you want using module in future, copy module into other folder (not into AndroidProjects folder and his subbfolders) and delete module permanetly from project and disc. Right mouse click on module and select from menu Delete.
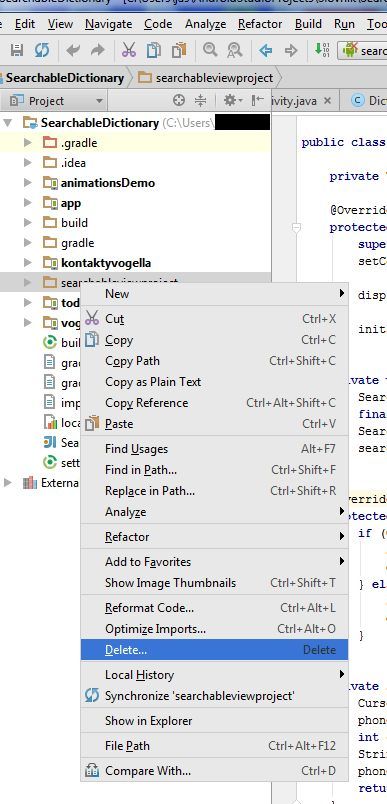
If you want import the backup copy to project, use: File>New>Import mudule
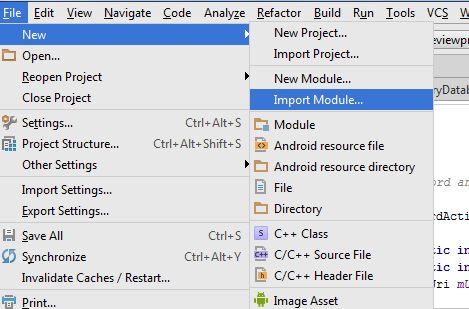
R.string to string Android source code
R.array to string [] Android source code
R.array to string [] Android source code
String s = getResources().getString(R.string.my_string); // Hello world!!
String[] arrayOfStrings = getResources().getStringArray(R.array.my_array); // one, two
<xml version="1.0" encoding="utf-8">
<resources>
<string-array name="my_array">
<item>one</item>
<item>two</item>
</string-array>
<string name="my_string">Hello world!!</string>
</resources>
Warning in AndroidManifest.xml:
tag should specify a target API level (the highest verified version; when running on
later versions, compatibility behaviors may be enabled) with android:targetSdkVersion="?"
Solution:
later versions, compatibility behaviors may be enabled) with android:targetSdkVersion="?"
Solution:
<uses-sdk android:minSdkVersion="4"
android:targetSdkVersion="16" />
Editace: 2011-09-26 20:52:49
Počet článků v kategorii: 396
Url:click-handler-android-code