Map TreeMap get values to array Java Android example
import java.util.Map;
import java.util.TreeMap;
public class MainClass {
public static void main(String[] arg) {
// english;germany dictionary
String[] arrayOfString = { "one;eine", "two;zwei", "two sets of;zwei"
, "three;drei", "four;vier" };
Map<String, String> map = new TreeMap<String, String>();
for (String s : arrayOfString) {
String[] array = s.split(";");
String sKey = "", sValue = "";
if (array.length > 1) {
sKey = array[0];
sValue = array[1];
map.put(sKey, sValue);
}
}
Object[] objectArrayOfValues = map.values().toArray();
for (int i = 0; i < objectArrayOfValues.length; i++) {
System.out.println(objectArrayOfValues[i]);
}
}// end main
}
/*
vier
eine
drei
zwei
zwei
*/
396LW NO topic_id
AD
Další témata ....(Topics)
public class MainClass extends Activity {
ActivityManager activityManager;
MemoryInfo memoryInfo;
final String TAG = "MemInfo";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
activityManager = (ActivityManager) getSystemService(ACTIVITY_SERVICE);
memoryInfo = new ActivityManager.MemoryInfo();
activityManager.getMemoryInfo(memoryInfo);
Log.i(TAG, " memoryInfo.availMem " + memoryInfo.availMem);
Log.i(TAG, " memoryInfo.lowMemory " + memoryInfo.lowMemory);
Log.i(TAG, " memoryInfo.threshold " + memoryInfo.threshold);
Toast.makeText(getApplicationContext(), String.valueOf( memoryInfo.availMem), Toast.LENGTH_LONG)
.show();
} // end onCreate
}
Brand | Samsung |
Model (codename) | Galaxy Y (S5360) |
Cena, včetně DPH | 2400 / 06.2012 |
Display size (v palcích) | 3 |
Display-resolution | 240x320 |
Dotek-typ | kapacitní |
CPU typ | BCM21553 |
CPU MHz | 832 |
CPU core | |
L2 cache | ? |
RAM | 256 |
ROM | 512 |
GPU | VideoCore IV |
NenaMark2 Benchmark | 12 |
GPU-GLBenchmark | |
Baterie mAh | 1200 |
Foto MPx | 2 |
Autofocus | ne |
Video | |
Official Android ICS | Android OS v 2.3 |
CyanogenMod support | |
Dotek-prstů-max | |
Display-ppi | 133 |
Display-retina | 41% |
Network | čîtyřpásmový GSM 850/900/1800/1900 MHz , HSDPA 2100 MHz |
Connectivity | Bluetooth v2.0 plus EDR plus support A2DP, USB host |
Pozn. | CPU ARMv6, GPU 20MT/s OpenGL ES 2.0 |
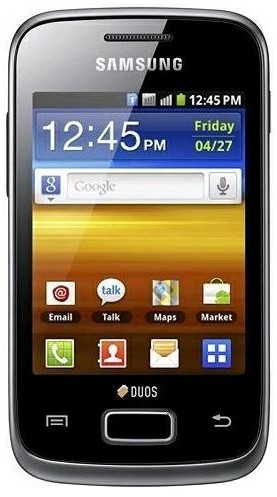
How include layout defined in other xml file into another xml file example:
res/layout/my_layout.xml into
main.xml
res/layout/my_layout.xml into
main.xml
<include layout="@layout/my_layout" android:id="@+id/idMyLayout" />
Bitmap Width, Height without memory allocation:
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeResource(getResources(), R.id.myimage, options);
// now opts.outWidth and opts.outHeight are the dimension of the
// bitmap, even though Bitmap is null
int imageHeight = options.outHeight;
int imageWidth = options.outWidth;
String imageType = options.outMimeType;
Nejlepší Smartphone jaro 2012.
Pdf file
Video - záznam a kvalita videa HTC One S
**VIDEO YOUTUBE
Brand | HTC |
---|---|
Model (codename) | One S |
Price - (Cena, včetně DPH) | 13000 |
Display size in Inch (v palcích) | 4.3 |
Display-resolution (rozlišení) | 960x540 |
Dotek-typ | multi-touch capacitive |
CPU typ | MSM8260A |
CPU MHz | 1500 |
CPU core | 2 |
L2 cache | |
ROM | 16 GB |
RAM | 1 GB |
GPU | Adreno 225 |
NenaMark2 Benchmark | |
GPU-GLBenchmark | |
Battery mAh | 1650 mAh, 10.5 hours of talk time and 13.2 days of stand-by time |
Foto MPx | 8 |
Autofocus | AF |
Video | HD záznam videa, 1920×1080 (1080p HD) @ 30 frame/s - 3GP, .3G2, MP4, WMV, AVI |
Official Android ICS | Android 4.0 Ice Cream Sandwich, HTC Sense 4.0 overlay |
CyanogenMod support | |
Dotek-prstů-max | |
Display-ppi | |
Display-retina | |
Network | HSPA/WCDMA: • Europe/Asia: 850/900/2100 MHz GSM/GPRS/EDGE: • 850/900/1800/1900 MHz |
Connectivity | 3.5 mm stereo audio jack Bluetooth with aptX™ enabled (Bluetooth® 4.0) Wi-Fi®: IEEE 802.11b/g/n DLNA® for wirelessly streaming media from the phone to a compatible TV or computer micro-USB 2.0 (5-pin) port with mobile high-definition video link (MHL) for USB or HDMI connection (Special cable required for HDMI connection.) |
Pozn. |
Pdf file
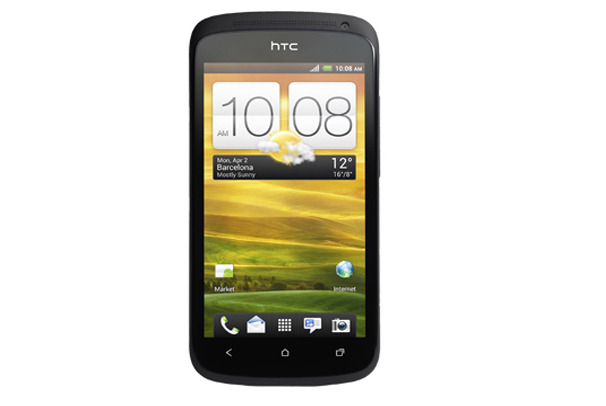
Video - záznam a kvalita videa HTC One S
**VIDEO YOUTUBE
Editace: 2013-12-09 13:29:16
Počet článků v kategorii: 396
Url:map-treemap-get-values-to-array-java-android-example