Hashtable key value pair add put get pair Java Android example
How add pair of strings to Hashtable, how get pair key value from Hashtable, how split string, basic Java Android example.
MainClass.java
MainClass.java
import java.util.Enumeration;
import java.util.Hashtable;
public class MainClass {
public static void main(String[] arg) {
// english;germany dictionary
String[] arrayOfString = { "one;eine", "two;zwei", "three;drei" };
Hashtable<String, String> hashTable = new Hashtable<String, String>();
for(String s: arrayOfString){
String[] array = s.split(";");
String sKey ="", sValue="";
if(array.length > 1){
sKey = array[0]; sValue = array[1];
hashTable.put(sKey, sValue);
}
}
Enumeration<String> enumer = hashTable.keys();
while (enumer.hasMoreElements()) {
String keyFromTable = (String) enumer.nextElement();
// get Returns the value to which the specified key is mapped,
// or null if this map contains no mapping for the key
System.out.println(keyFromTable + " = " + hashTable.get(keyFromTable));
}
}
}
/*
two = zwei
one = eine
three = drei
*/
396LW NO topic_id
AD
Další témata ....(Topics)
Tutoriál je pro naprosté začátečníky s fragmenty, machři se nic nového nedozvědí.
Tutoriál se bude zabývat upozorněním na některé záludnosti v příkladu, jenž si pozorně pročtěte, a
který si můžete otevřít a stáhnout zde:
https://developer.android.com/training/basics/fragments/creating.html
Je tam i zip soubor, který si stáhněte a otevřete v Android Studiu (JetBrains IntelliJ IDEA software),
či jiném IDE, které používáte k programování.
Začneme soubory XML
V příkladu jsou v res složce dvě složky layout.
layout - pro obrazovky chytrých telefonů a
layout-large - pro obrazovky tabletů a větších obrazovek
V složce layout jsou dva soubory.
article_view.xml je v tomto případě vždy využíván fragmentem ArticleFragment.java, který zobrazuje obsah. Je jedno jaká bude velikost obrazovky, protože si jej otevírá ArticleFragment.java sám.
Obě složky obsahují soubor stejného názvu news_articles.xml - který obsahuje kontejner buď jen pro jeden panel (layout složka),
nebo pro dva panely (layout-large složka) pro velké obrazovky.
news_articles.xml v layout složce obsahuje jen FrameLayout
news_articles.xml v layout-large složce obsahuje dva kontainery tagu fragment s plnou cestou k souboru např. com.example.android.fragments.HeadlinesFragment,
u kterých není možná dynamická výměna fragmentu!!!!
Tutoriál se bude zabývat upozorněním na některé záludnosti v příkladu, jenž si pozorně pročtěte, a
který si můžete otevřít a stáhnout zde:
https://developer.android.com/training/basics/fragments/creating.html
Je tam i zip soubor, který si stáhněte a otevřete v Android Studiu (JetBrains IntelliJ IDEA software),
či jiném IDE, které používáte k programování.
Important: Protože Android Studio má celkem dost značné nároky na PC, zejména na rychlost a budete si chtít pořídit nové PC, je třeba s procesorem Intel a nekupovat repas, ale vše v novotě. Doporučované minimum je nedostatečné a práce na takovém PC je horor. Dole na stránce odkazu je uveden typ procesoru, který by mělo PC mít, jinak na něm nespustíte, nebo jen s obtížemi, emulátor, na kterém se testují vytvářené aplikace.
Například pro rok 2016 jsou požadavky na procesor:
For accelerated emulator: 64-bit operating system and Intel® processor with support for Intel® VT-x, Intel® EM64T (Intel® 64), and Execute Disable (XD) Bit functionality
Začneme soubory XML
V příkladu jsou v res složce dvě složky layout.
layout - pro obrazovky chytrých telefonů a
layout-large - pro obrazovky tabletů a větších obrazovek
V složce layout jsou dva soubory.
article_view.xml je v tomto případě vždy využíván fragmentem ArticleFragment.java, který zobrazuje obsah. Je jedno jaká bude velikost obrazovky, protože si jej otevírá ArticleFragment.java sám.
Obě složky obsahují soubor stejného názvu news_articles.xml - který obsahuje kontejner buď jen pro jeden panel (layout složka),
nebo pro dva panely (layout-large složka) pro velké obrazovky.
Important:
Složku layout-large lze přejmenovat na swXXXdp např. sw600dp a pak si zařízení bere automaticky resource z této složky, pokud nejmenší rozměr obrazovky je roven, či větší 600dp.
To lze využít k oklamání zařízení při testování. Pokud máte jen malý telefon a chcete na něm zkoušet dva panely, tak složku přejmenujte, po dobu testování, např. na sw300dp a pak i malé zařízení zobrazí oba panely. Po ukončení testování je třeba zase složku přejmenovat na původní nejmenší přípustnou šířku zařízení (w600dp), nebo nejmenší rozměr jedné ze stran obrazovky (sw600dp).
news_articles.xml v layout složce obsahuje jen FrameLayout
<FrameLayout xmlns:android="//schemas.android.com/apk/res/android"
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Important: U tohoto kontaineru -FrameLayout- je možno měnit obsah, tedy dynamicky vyměnit fragment za jiný! Toho využívá aplikace u malých obrazovek pro zobrazení jak seznamu, tak i dalšího obsahu po kliknutí na položku v ListView - seznamu.
U fragment kontaineru s natvrdo přiřazeným fragmentem dynamická výměna za běhu programu není možná (viz níže dva kontainery tagu fragment)!!!
news_articles.xml v layout-large složce obsahuje dva kontainery tagu fragment s plnou cestou k souboru např. com.example.android.fragments.HeadlinesFragment,
u kterých není možná dynamická výměna fragmentu!!!!
Important: Pokud chcete měnit fragmenty v některém kontaineru, je třeba použít FrameLayout kontainer!!!!
<fragment android:name="com.example.android.fragments.HeadlinesFragment"
android:id="@+id/headlines_fragment"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="match_parent" />
<fragment android:name="com.example.android.fragments.ArticleFragment"
android:id="@+id/article_fragment"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent" />
Important: Na co si ještě dát pozor!!!!
Na android:layout_width="0dp" - musí být 0dp, jinak se např. některý panel nezobrazí, nebo neuvídíte vůbec nic.
Podobně i android:layout_weight="1" u prvního panelu android
android:layout_weight="2" u panelu druhého!!!!!
Canvas, drawLine(), setStrokeWidth(), Paint, setAntiAlias(boolean), onDraw()
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
canvas.drawColor(Color.YELLOW);
Paint p = new Paint();
// smooths
p.setAntiAlias(true);
p.setColor(Color.RED);
p.setStrokeWidth(4.5f);
// opacity
p.setAlpha(0x80); //
// drawLine (float startX, float startY, float stopX, float stopY,
// Paint paint)
canvas.drawLine(0, 0, 40, 40, p);
canvas.drawLine(40, 0, 0, 40, p);
}
}
}
Unable to resolve target android-7
Try this solution:
Select project from tree (project explorer)
- right click on project
- properties
- select Android from tree
- change Project Build Target to higher (or change project build target)
- selct from menu: Project-Clean ( select your project - OK)
Try this solution:
Select project from tree (project explorer)
- right click on project
- properties
- select Android from tree
- change Project Build Target to higher (or change project build target)
- selct from menu: Project-Clean ( select your project - OK)
choreographer skipped frames
Create filter with TAG regex to disable Choreographer messages, see code and picture below:
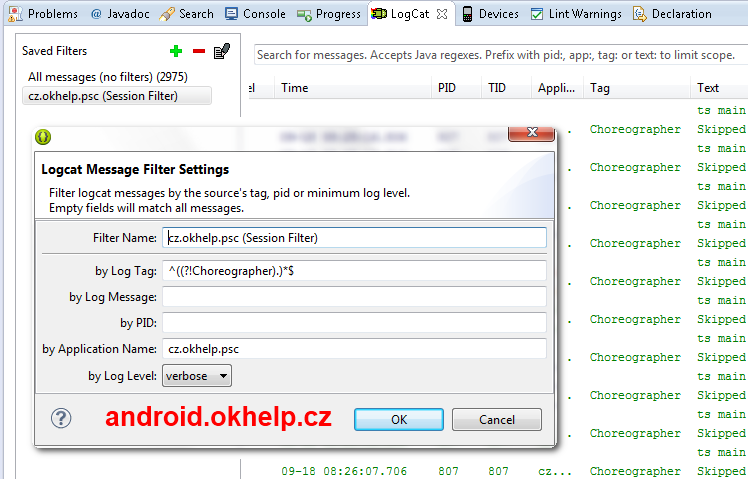
Create filter with TAG regex to disable Choreographer messages, see code and picture below:
^((?!Choreographer).)*$
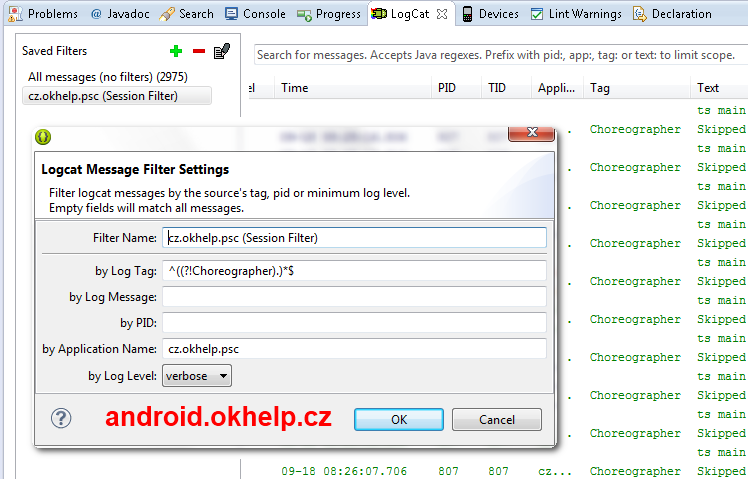
Warning: The application may be doing too much work on its main thread
Try this sorce code:
Try this sorce code:
import android.os.StrictMode;
public class MyActivity extends Activity {
static{
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
@Override
public void onCreate(Bundle savedInstanceState) {
//.................. etc.
Editace: 2013-12-09 10:56:37
Počet článků v kategorii: 396
Url:hashtable-key-value-pair-add-put-get-pair-java-android-example