Rotate a image bitmap picture Android example
Rotate a bitmap Android source code.
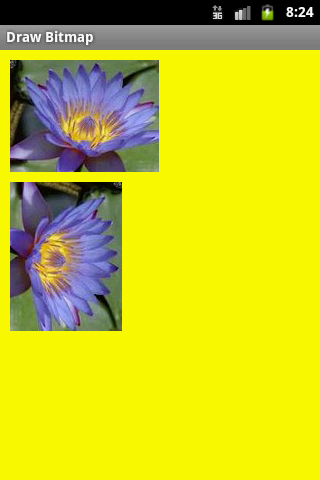
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
canvas.drawColor(Color.YELLOW);
// Bitmap b = Bitmap.createBitmap(200, 200, Bitmap.Config.ARGB_8888);
// you need to insert a image flower_blue into res/drawable folder
Bitmap bmp = BitmapFactory.decodeResource(getResources(), R.drawable.flower_blue);
Matrix mat = new Matrix();
mat.postRotate(90);
Bitmap bmpRotate = Bitmap.createBitmap(bmp, 0, 0,
bmp.getWidth(), bmp.getHeight(),
mat, true);
int h = bmp.getHeight();
canvas.drawBitmap(bmp, 10,10, paint);
canvas.drawBitmap(bmpRotate, 10,10 + h + 10, paint);
}
}
}
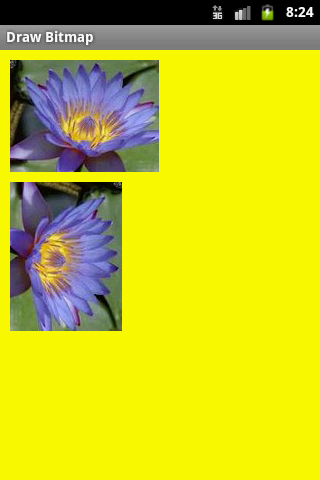
396LW NO topic_id
AD
Další témata ....(Topics)
Show keyboard Android phone apps development example source code.
// ActivityClass.java
InputMethodManager showSoftInput;
Button hBtnKeyboardShow ;
//onCreate
showSoftInput = (InputMethodManager)this.getSystemService(Context.INPUT_METHOD_SERVICE);
hBtnKeyboardShow = (Button)findViewById(R.id.btnKeyboardShow);
hBtnKeyboardShow.setOnClickListener(myButtonListener);
// END onCreate
//button listener
private OnClickListener myButtonListener = new OnClickListener() {
public void onClick(View v) {
try {
showSoftInput.getInputMethodList();
showSoftInput.toggleSoftInput(showSoftInput.SHOW_FORCED, 0);
} catch (Exception e) {
Log.e("Keyboard show ", e.getMessage());
}
}
};
Android development example source code
Get supported language:
// import
import android.speech.tts.TextToSpeech;
import android.speech.tts.TextToSpeech.OnInitListener;
// you have to add implementation
public class Main extends Activity implements TextToSpeech.OnInitListener {
private int _langTTSavailable = -1; // set up in onInit method
// declaration
private TextToSpeech mTts;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// assigned handle - initialisation
mTts = new TextToSpeech(this,
(OnInitListener) this // TextToSpeech.OnInitListener
);
}
// Implements TextToSpeech.OnInitListener.
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
// Set preferred language to US english.
_langTTSavailable = mTts.setLanguage(Locale.US); // Locale.FRANCE etc.
if (_langTTSavailable == TextToSpeech.LANG_MISSING_DATA ||
_langTTSavailable == TextToSpeech.LANG_NOT_SUPPORTED) {
} else if ( _langTTSavailable >= 0) {
mTts.speak("Good morning",
TextToSpeech.QUEUE_FLUSH, // Drop all pending entries in the playback queue.
null);
}
} else {
// Initialization failed.
}
}
@Override
public void onDestroy() {
// TTS shutdown!
if (mTts != null) {
mTts.stop();
mTts.shutdown();
}
super.onDestroy();
}
}
Get supported language:
private TextToSpeech mTts;
// public void onInit(int status){
int result;
String s;
result = mTts.setLanguage( Locale. CANADA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CANADA not supported<br>" ;}else{s+=" CANADA supported<br>";}
result = mTts.setLanguage( Locale. CANADA_FRENCH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CANADA_FRENCH not supported<br>" ;}else{s+=" CANADA_FRENCH supported<br>";}
result = mTts.setLanguage( Locale. CHINA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CHINA not supported<br>" ;}else{s+=" CHINA supported<br>";}
result = mTts.setLanguage( Locale. CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " CHINESE not supported<br>" ;}else{s+=" CHINESE supported<br>";}
result = mTts.setLanguage( Locale. ENGLISH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ENGLISH not supported<br>" ;}else{s+=" ENGLISH supported<br>";}
result = mTts.setLanguage( Locale. FRANCE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " FRANCE not supported<br>" ;}else{s+=" FRANCE supported<br>";}
result = mTts.setLanguage( Locale. FRENCH ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " FRENCH not supported<br>" ;}else{s+=" FRENCH supported<br>";}
result = mTts.setLanguage( Locale. GERMAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " GERMAN not supported<br>" ;}else{s+=" GERMAN supported<br>";}
result = mTts.setLanguage( Locale. GERMANY ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " GERMANY not supported<br>" ;}else{s+=" GERMANY supported<br>";}
result = mTts.setLanguage( Locale. ITALIAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ITALIAN not supported<br>" ;}else{s+=" ITALIAN supported<br>";}
result = mTts.setLanguage( Locale. ITALY ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ITALY not supported<br>" ;}else{s+=" ITALY supported<br>";}
result = mTts.setLanguage( Locale. JAPAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " JAPAN not supported<br>" ;}else{s+=" JAPAN supported<br>";}
result = mTts.setLanguage( Locale. JAPANESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " JAPANESE not supported<br>" ;}else{s+=" JAPANESE supported<br>";}
result = mTts.setLanguage( Locale. KOREA ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " KOREA not supported<br>" ;}else{s+=" KOREA supported<br>";}
result = mTts.setLanguage( Locale. KOREAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " KOREAN not supported<br>" ;}else{s+=" KOREAN supported<br>";}
result = mTts.setLanguage( Locale. PRC ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " PRC not supported<br>" ;}else{s+=" PRC supported<br>";}
result = mTts.setLanguage( Locale. ROOT ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " ROOT not supported<br>" ;}else{s+=" ROOT supported<br>";}
result = mTts.setLanguage( Locale. SIMPLIFIED_CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " SIMPLIFIED_CHINESE not supported<br>" ;}else{s+=" SIMPLIFIED_CHINESE supported<br>";}
result = mTts.setLanguage( Locale. TAIWAN ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " TAIWAN not supported<br>" ;}else{s+=" TAIWAN supported<br>";}
result = mTts.setLanguage( Locale. TRADITIONAL_CHINESE ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " TRADITIONAL_CHINESE not supported<br>" ;}else{s+=" TRADITIONAL_CHINESE supported<br>";}
result = mTts.setLanguage( Locale. UK ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " UK not supported<br>" ;}else{s+=" UK supported<br>";}
result = mTts.setLanguage( Locale. US ); if (result == TextToSpeech.LANG_MISSING_DATA ||result == TextToSpeech.LANG_NOT_SUPPORTED) {s += " US not supported<br>" ;}else{s+=" US supported<br>";}
Code with error:
If you get error try this code:
java.lang.ClassCastException: android.view.ViewGroup$LayoutParams
at android.widget.LinearLayout.measureHorizontal(LinearLayout.java:659)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:311)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.LinearLayout.measureChildBeforeLayout(LinearLayout.java:1017)
at android.widget.LinearLayout.measureVertical(LinearLayout.java:386)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:309)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.FrameLayout.onMeasure(FrameLayout.java:250)
at android.view.View.measure(View.java:8313)
at android.widget.LinearLayout.measureVertical(LinearLayout.java:531)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:309)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.FrameLayout.onMeasure(FrameLayout.java:250)
at android.view.View.measure(View.java:8313)
ImageView imgV = (ImageView)findViewById(R.id.myView);
imgV.setLayoutParams(new ViewGroup.LayoutParams(
ViewGroup.LayoutParams.WRAP_CONTENT,
0));
If you get error try this code:
ViewGroup.LayoutParams layoutParams = imgV
.getLayoutParams();
layoutParams.height = 0;
imgV.setLayoutParams(layoutParams);
java.lang.ClassCastException: android.view.ViewGroup$LayoutParams
at android.widget.LinearLayout.measureHorizontal(LinearLayout.java:659)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:311)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.LinearLayout.measureChildBeforeLayout(LinearLayout.java:1017)
at android.widget.LinearLayout.measureVertical(LinearLayout.java:386)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:309)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.FrameLayout.onMeasure(FrameLayout.java:250)
at android.view.View.measure(View.java:8313)
at android.widget.LinearLayout.measureVertical(LinearLayout.java:531)
at android.widget.LinearLayout.onMeasure(LinearLayout.java:309)
at android.view.View.measure(View.java:8313)
at android.view.ViewGroup.measureChildWithMargins(ViewGroup.java:3138)
at android.widget.FrameLayout.onMeasure(FrameLayout.java:250)
at android.view.View.measure(View.java:8313)
TypeFace, setTypeface, font, font family, array of String, Button create dynamically
@Override
protected void onStart() {
LinearLayout.LayoutParams p = new LinearLayout.LayoutParams(
48,
55
);
Typeface typeFace = Typeface.MONOSPACE;
String [] ar = {"A","B","C","D","E","F","G","H","J","K"};
for (int i = 0; i < ar.length; i++) {
Button buttonView = new Button(this);
buttonView.setText(Html.fromHtml("<b>"+ar[i]+"</b>"));
buttonView.setTextColor(Color.BLUE);
buttonView.setTextSize(27.f);
buttonView.setTypeface(typeFace,Typeface.BOLD);
buttonView.setOnClickListener(mThisButtonListener);
if(i%2==0)
mLayoutButtons.addView(buttonView, p);
else
mLayoutButtonsNextRow.addView(buttonView, p);
_listOfButtons.add(buttonView);
}
super.onStart();
}
//////// xml file
<TextView
android:id="@+id/text01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
android:fontFamily="Arial"
/>
public class Main extends Activity {
private TextView mTextView;
private Activity mAct;
private Intent mIntent;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_layout);
mTextView = findViewById(R.id.mTextView);
mAct = getActivity();
mIntent = getIntent();
}
}
to:
public class Main extends Fragment{
private TextView mTextView;
private FragmentActivity mFrgAct;
private Intent mIntent;
private LinearLayout mLinearLayout;
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View root = inflater.inflate(R.layout.fragment_main, null);
return root;
}
public void onViewCreated(View view, Bundle savedInstanceState) {
// you can add listener of elements here
/*Button mButton = (Button) view.findViewById(R.id.button);
mButton.setOnClickListener(this); */
mTextView = view.findViewById(R.id.mTextView);
mLinearLayout = (LinearLayout)view;
}
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
mFrgAct = getActivity();
mIntent = mFrgAct.getIntent(); // Intent intent = new Intent(getActivity().getIntent());
}
}
Editace: 2011-11-15 07:01:41
Počet článků v kategorii: 396
Url:rotate-a-image-bitmap-android-example