List LinkedList add sort find Item in Java example
List LinkedList Collections add sort find search Item, get searched item to string in Java example.
MainClass.java
MainClass.java
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = {"nothing", "Hello", "people"
, "bye-bye", "hello", "world!", "End" };
List<String> arrayList = new LinkedList<String>();
for(String s: arrayOfString)
arrayList.add(s);
Collections.sort(arrayList);
// foreach
for (String str: arrayList)
System.out.println(str);
Object objMin = Collections.min(arrayList);
System.out.println("Min is: " + objMin);
Object objMax = Collections.max(arrayList);
System.out.println("Max is: " + objMax);
int index = Collections.binarySearch(arrayList, "people");
System.out.println("Index of people is: " + index);
// print word on index 5
System.out.println("Index 5 is: " + arrayList.get(5));
String sItem = arrayList.get(5); // get item from index 5 to string
}
}
/*
End
Hello
bye-bye
hello
nothing
people
world!
Min is: End
Max is: world!
Index of people is: 5
Index 5 is: people
*/
396LW NO topic_id
AD
Další témata ....(Topics)
// solution 1
ImageView imageView = (ImageView)findViewById(R.id.myimage);
imageView.setImageDrawable(null);
// or
imageView.setImageResource(0);
// solution 2 hide ImageView
imageView.setVisibility(View.INVISIBLE);
// solution 3 resize ImageView 0, 0
hImageViewSemafor.setLayoutParams(new LinearLayout.LayoutParams(0,0));
Try this solution:
String DATA = "Html text....bla bla bla. Hellou world! čšřžěéá";
String HEADERHTML =
"<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">"
+"<html> <head> <meta http-equiv="content-type" content="text/html; charset=utf-8">"
+"</head> <body>";
String FOOTERHTML = "</body></html>";
WebView mWebView.loadData(HEADERHTML+DATA+FOOTERHTML,"text/html; charset=UTF-8",null);
Switch statement with numbers and array of strings Java example.
Possible:
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "One", "Two", "Three", "Four" };
int i = 2;
switch (i) {
case 1: {
System.out.println(arrayOfString[i]);
break;
}
case 2: {
System.out.println(arrayOfString[i]);
break;
}
case 3: {
System.out.println(arrayOfString[i]);
break;
}
default: {
System.out.println("Enter a valid value.");
}
} // END of switch
}
}
/*
* Three
*/
Possible:
case 1:
System.out.println(arrayOfString[i]);
break;
// i love this notation
case 1:{
System.out.println(arrayOfString[i]);
}break;
case 1:{
System.out.println(arrayOfString[i]);
break;
}
While cycle Java basic example with array of strings.
MainClass.java
MainClass.java
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "Hello", "people", "hello", "world!" };
int i = 0;
while ( i < arrayOfString.length ){
System.out.println(arrayOfString[i]);
i++;
}
}
}
onSaveInstanceState, onRestoreInstanceState life cycle if screen orientation changed from log file.
Diagram of life cycle onSaveInstanceState, onRestoreInstanceState
[caption id="attachment_1169" align="alignleft" width="229" caption="Life cycle onRestoreInstanceState"]
[/caption]
// starts activity
15:27:12.801: INFO/onCreate(1828): onCreate()
15:27:12.811: INFO/onStart(1828): onStart()
15:27:12.821: INFO/onResume(1828): onResume()
// activity is running
15:27:33.651: DEBUG/dalvikvm(307): GC_EXPLICIT freed 186K, 53%
free 2770K/5831K, external 981K/1038K, paused 99ms
// change emulator state Ctrl+F11 landscape, portrait
15:27:40.427: INFO/ActivityManager(74): Config changed:
{ scale=1.0 imsi=310/260 loc=en_US touch=3 keys=2/1/2 nav=3/1 orien=2 layout=18 uiMode=17 seq=64}
// saved all variable values if need
15:27:40.581: INFO/onSaveInstanceState(1828): onSaveInstanceState()
15:27:40.602: INFO/onPause(1828): onPause()
15:27:40.612: INFO/onStop(1828): onStop()
15:27:40.631: INFO/onDestroy(1828): onDestroy()
// activity goes back to onCreate !!!!!!!!!
15:27:40.692: INFO/onCreate(1828): onCreate()
15:27:40.701: INFO/onStart(1828): onStart()
// restore all saved values of variables
15:27:40.711: INFO/onRestoreInstanceState(1828): onRestoreInstanceState()
// you can using saved values by onSaveInstanceState() in onResume
15:27:40.721: INFO/onResume(1828): onResume()
Diagram of life cycle onSaveInstanceState, onRestoreInstanceState
[caption id="attachment_1169" align="alignleft" width="229" caption="Life cycle onRestoreInstanceState"]
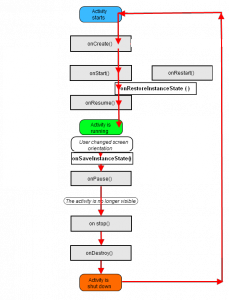
Editace: 2013-12-09 10:56:55
Počet článků v kategorii: 396
Url:list-linkedlist-add-sort-find-item-in-java-example