AsyncTask Example Android with ProgressBar
MainActivity.java
activity_main.xml
AndroidManifest.xml do not forget INTERNET uses-permission !!!!!!!
package com.asynctaskexample;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import android.os.AsyncTask;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.ProgressBar;
import android.widget.TextView;
public class MainActivity extends Activity {
private TextView textView;
private ProgressBar progressBar;
private DownloadWebPageTask mTask = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = (TextView) findViewById(R.id.TextView01);
downloadPage();
}
// AsyncTask <TypeOfVarArgParams , ProgressValue , ResultValue> .
private class DownloadWebPageTask extends AsyncTask<String, Integer, String> {
@Override
protected void onPreExecute() {
//textView.setText("Hello !!!");
progressBar = (ProgressBar) findViewById(R.id.progressBar1);
progressBar.setVisibility(View.VISIBLE);
super.onPreExecute();
}
@Override
protected void onProgressUpdate(Integer... values) {
super.onProgressUpdate(values);
}
@Override
protected String doInBackground(String... urls) {
String response = "";
for (String url : urls) {
DefaultHttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse execute = client.execute(httpGet);
InputStream content = execute.getEntity().getContent();
BufferedReader buffer = new BufferedReader(new InputStreamReader(
content));
String s = "";
while ((s = buffer.readLine()) != null) {
response += s;
}
} catch (Exception e) {
e.printStackTrace();
}
}
return response;
}
@Override
protected void onPostExecute(String result) {
progressBar.setVisibility(View.INVISIBLE);
textView.setText(result);
}
}
private void downloadPage() {
if (mTask != null
&& mTask.getStatus() != DownloadWebPageTask.Status.FINISHED) {
mTask.cancel(true);
}
// execute(String[]) you can put array of links to web pages, or array of Integer[]
// if first param is Integer[] etc.
mTask = (DownloadWebPageTask) new DownloadWebPageTask()
.execute(new String[] { "//android.okhelp.cz/android-market.html",
"//android.okhelp.cz/android-market.html" });
}
@Override
protected void onDestroy() {
super.onDestroy();
if (mTask != null
&& mTask.getStatus() != DownloadWebPageTask.Status.FINISHED) {
mTask.cancel(true);
mTask = null;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
activity_main.xml
<LinearLayout xmlns:android="//schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ProgressBar
android:id="@+id/progressBar1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/TextView01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world" />
</LinearLayout>
AndroidManifest.xml do not forget INTERNET uses-permission !!!!!!!
<uses-permission android:name="android.permission.INTERNET" />
396LW NO topic_id
AD
Další témata ....(Topics)
Locale lc = Locale.getDefault(); // default now locale on device
String sCountry = lc.getCountry(); // CZ
lc = new Locale("fr","FR"); //FRANCE .. Locale(language, country);
String sCountry2 = lc.getDisplayCountry(); // Francie
Locale locale = Locale.GERMAN;
String sCountry3 = locale.getDisplayCountry(); // ""
Locale locale = Locale.GERMAN;
DateFormat formatter = new SimpleDateFormat("HH:mm:ss zzzz", locale);
String s = formatter.format(new Date());//13:40:39 GMT+00:00
// array of locales
Locale[] locales = { new Locale("fr", "FR"), new Locale("de", "DE"),
new Locale("en", "US") };
Locale locale = Locale.US;
// for date
DateFormat dateFormatterEurope = DateFormat.getDateInstance(DateFormat.DEFAULT,
Locale.GERMANY);
Calendar myCalendar = Calendar.getInstance();
String sDate = dateFormatterEurope.format(myCalendar.getTime());
final byte[] langBytes = locale.getLanguage().getBytes(Charsets.US_ASCII);
// UTF-8 most widely used text format for to properly display of text
final Charset utfEncoding = Charsets.UTF_8;
String text = "ěščřžýáíéůú";
final byte[] textBytes = text.getBytes(utfEncoding);
// other Locale
CANADA
CANADA_FRENCH
CHINA
CHINESE
ENGLISH
FRANCE
FRENCH
GERMAN
GERMANY
ITALIAN
ITALY
JAPAN
JAPANESE
KOREA
KOREAN
PRC // Locale constant for zh_CN.
ROOT // Locale constant for the root locale.
SIMPLIFIED_CHINESE
TAIWAN
TRADITIONAL_CHINESE Locale constant for zh_TW.
UK
US
public class ApokusActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
canvas.drawColor(Color.YELLOW);
paint.setFilterBitmap(true);
Bitmap bitmapOrg = BitmapFactory.decodeResource(getResources(),R.drawable.flower_blue);
int targetWidth = bitmapOrg.getWidth() * 2;
int targetHeight = bitmapOrg.getHeight() * 2;
Bitmap bmp = Bitmap.createBitmap(targetWidth, targetHeight,Bitmap.Config.ARGB_8888);
RectF rectf = new RectF(0, 0, targetWidth, targetHeight);
Canvas c = new Canvas(bmp);
Path path = new Path();
path.addRect(rectf, Path.Direction.CW);
c.clipPath(path);
c.drawBitmap( bitmapOrg, new Rect(0, 0, bitmapOrg.getWidth(), bitmapOrg.getHeight()),
new Rect(0, 0, targetWidth, targetHeight), paint);
Matrix matrix = new Matrix();
matrix.postScale(1f, 1f);
Bitmap resizedBitmap = Bitmap.createBitmap(bmp, 0, 0, targetWidth, targetHeight, matrix, true);
int h = bitmapOrg.getHeight();
canvas.drawBitmap(bitmapOrg, 10,10, paint);
canvas.drawBitmap(resizedBitmap, 10,10 + h + 10, paint);
}
}
}
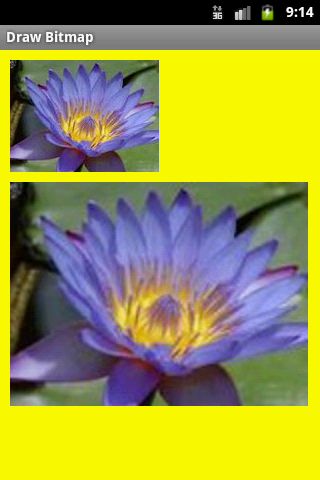
InputStream, getResources(),openRawResource()
java.io.InputStream is;
is = context.getResources().openRawResource(R.drawable.my_image);
Drawable mDrawable = context.getResources().getDrawable(R.drawable.button);
mDrawable.setBounds(150, 20, 300, 100);
Drawable[] mDrawables;
int[] resIDs = new int[] {
R.drawable.btn_ok,
R.drawable.btn_storno,
R.drawable.btn_help
};
mDrawables = new Drawable[resIDs.length];
Drawable prev = mDrawable;
for (int i = 0; i < resIDs.length; i++) {
mDrawables[i] = context.getResources().getDrawable(resIDs[i]);
mDrawables[i].setDither(true);
addToTheRight(mDrawables[i], prev);
prev = mDrawables[i];
}
choreographer skipped frames
Create filter with TAG regex to disable Choreographer messages, see code and picture below:
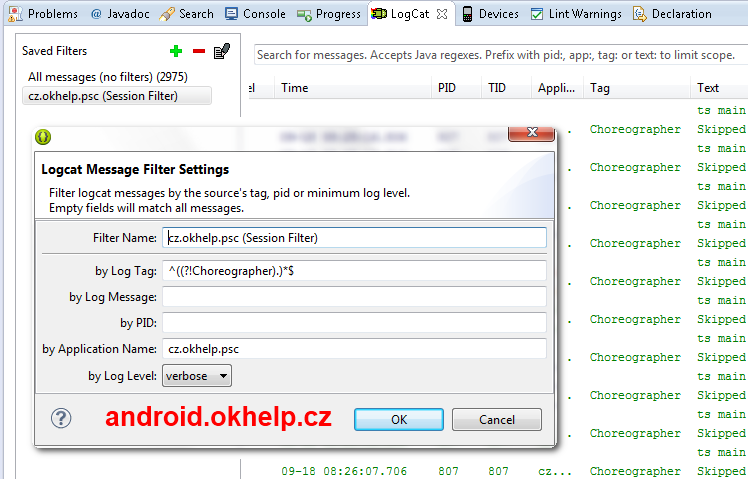
Create filter with TAG regex to disable Choreographer messages, see code and picture below:
^((?!Choreographer).)*$
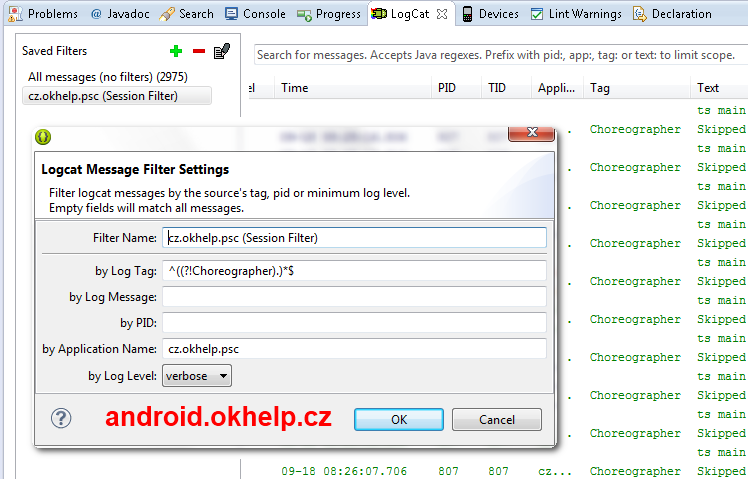
Convert number int to string Android Java example source code.
int n = 0;
try {
n = Integer.parseInt("21"));
} catch(NumberFormatException e) {
System.out.println("Could not parse " + e);
}
// int to string
String s = String.valueOf(24);
Editace: 2012-12-27 15:30:36
Počet článků v kategorii: 396
Url:asynctask-example-android-with-progressbar