Programmatically determine if App is running on Android Emulator
You can get list by class Build
For example:
List of Build class getting from emulator:
"BOARD=unknown
BRAND=generic
CPU_ABI=armeabi
DEVICE=generic
DISPLAY=sdk-eng 2.1-update1 ECLAIR 35983 test-keys
FINGERPRINT=generic/sdk/generic/:2.1-update1/ECLAIR/35983:eng/test-keys
HOST=android-test-13.mtv.corp.google.com
ID=ECLAIR
MANUFACTURER=unknown
MODEL=sdk
PRODUCT=sdk
TAGS=test-keys
TIME=1273161972000
TYPE=eng
USER=android-build
"
For example:
if(Build.MANUFACTURER.equals("unknown")) {
// Emulator
}
List of Build class getting from emulator:
"BOARD=unknown
BRAND=generic
CPU_ABI=armeabi
DEVICE=generic
DISPLAY=sdk-eng 2.1-update1 ECLAIR 35983 test-keys
FINGERPRINT=generic/sdk/generic/:2.1-update1/ECLAIR/35983:eng/test-keys
HOST=android-test-13.mtv.corp.google.com
ID=ECLAIR
MANUFACTURER=unknown
MODEL=sdk
PRODUCT=sdk
TAGS=test-keys
TIME=1273161972000
TYPE=eng
USER=android-build
"
396LW NO topic_id
AD
Další témata ....(Topics)
Try add to AndroidManifest.xml configChanges for your Activity
android:configChanges="keyboardHidden|orientation|screenSize"
android:configChanges="keyboardHidden|orientation|screenSize"
<activity android:name=".MainActivity"
android:label="@string/app_name"
android:configChanges="keyboardHidden|orientation|screenSize"
>
public class ApokusActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
canvas.drawColor(Color.YELLOW);
paint.setFilterBitmap(true);
Bitmap bitmapOrg = BitmapFactory.decodeResource(getResources(),R.drawable.flower_blue);
int targetWidth = bitmapOrg.getWidth() * 2;
int targetHeight = bitmapOrg.getHeight() * 2;
Bitmap bmp = Bitmap.createBitmap(targetWidth, targetHeight,Bitmap.Config.ARGB_8888);
RectF rectf = new RectF(0, 0, targetWidth, targetHeight);
Canvas c = new Canvas(bmp);
Path path = new Path();
path.addRect(rectf, Path.Direction.CW);
c.clipPath(path);
c.drawBitmap( bitmapOrg, new Rect(0, 0, bitmapOrg.getWidth(), bitmapOrg.getHeight()),
new Rect(0, 0, targetWidth, targetHeight), paint);
Matrix matrix = new Matrix();
matrix.postScale(1f, 1f);
Bitmap resizedBitmap = Bitmap.createBitmap(bmp, 0, 0, targetWidth, targetHeight, matrix, true);
int h = bitmapOrg.getHeight();
canvas.drawBitmap(bitmapOrg, 10,10, paint);
canvas.drawBitmap(resizedBitmap, 10,10 + h + 10, paint);
}
}
}
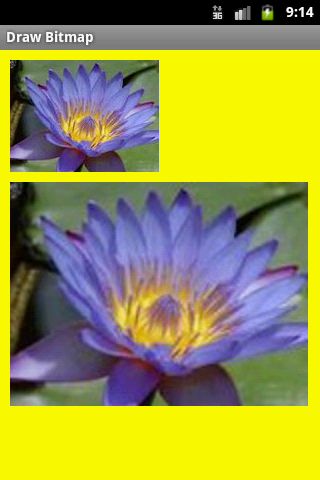
Brand | Samsung |
Model (codename) | i9250 Galaxy Nexus |
Cena, včetně DPH | 9000 |
Veikost Displaye v palcích | 4.65 |
Display-resolution | 1280x720 |
Dotek-typ | kapacitní |
CPU typ | |
CPU MHz | 1200 |
CPU core | 2 |
L2 cache | |
RAM | 1 GB |
ROM | 16 - 32 GB |
GPU | TI OMAP 4460 1,2 GHz dual-core |
NenaMark2 Benchmark | |
GPU-GLBenchmark | |
Baterie mAh | 1750 |
Foto MPx | 5 |
Autofocus | yes |
Video | 480p - 30 frames/s |
Official Android ICS | Android 4.0 Ice Cream Sandwich |
CyanogenMod support | |
Dotek-prstů-max | |
Display-ppi | |
Display-retina | |
Network | GSM&EDGE: 850 / 900 / 1.800 / 1.900 |
Connectivity | |
Pozn. |
samsung-i9250-galaxy-nexus image
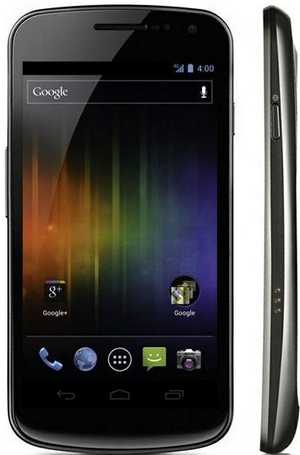
Canvas, drawLine(), setStrokeWidth(), Paint, setAntiAlias(boolean), onDraw()
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
canvas.drawColor(Color.YELLOW);
Paint p = new Paint();
// smooths
p.setAntiAlias(true);
p.setColor(Color.RED);
p.setStrokeWidth(4.5f);
// opacity
p.setAlpha(0x80); //
// drawLine (float startX, float startY, float stopX, float stopY,
// Paint paint)
canvas.drawLine(0, 0, 40, 40, p);
canvas.drawLine(40, 0, 0, 40, p);
}
}
}
Foreach in Java basic example source code.
MainClass.java
MainClass.java
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "Hello", "people", "hello", "world!" };
for (String s : arrayOfString)
System.out.println(s);
}
}
Editace: 2012-12-12 19:47:42
Počet článků v kategorii: 396
Url:programmatically-determine-if-app-is-running-on-android-emulator