Bitmap Canvas Color Gradient linear radial sweep rounded corner Android example
public class MainActivity extends Activity {
// //www.apache.org/licenses/LICENSE-2.0
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
private Rect mRect;
private GradientDrawable mDrawable;
public SampleView(Context context) {
super(context);
setFocusable(true);
mRect = new Rect(0, 0, 220, 120);
/* GradientDrawable.Orientation BL_TR draw the gradient from the bottom-left to the top-right
BOTTOM_TOP draw the gradient from the bottom to the top
BR_TL draw the gradient from the bottom-right to the top-left
LEFT_RIGHT draw the gradient from the left to the right
RIGHT_LEFT draw the gradient from the right to the left
TL_BR draw the gradient from the top-left to the bottom-right
TOP_BOTTOM draw the gradient from the top to the bottom
TR_BL draw the gradient from the top-right to the bottom-left
*/
mDrawable = new GradientDrawable(GradientDrawable.Orientation.LEFT_RIGHT,
new int[] { 0xFFFF0000, 0xFF00FF00,
0xFF0000FF });
mDrawable.setShape(GradientDrawable.RECTANGLE);
mDrawable.setGradientRadius((float)(Math.sqrt(2) * 60));
}
static void setCornerRadius(GradientDrawable drawable, float r0,
float r1, float r2, float r3) {
/* setCornerRadii
Specify radii for each of the 4 corners. For each corner,
the array contains 2 values, [X_radius, Y_radius].
The corners are ordered top-left, top-right, bottom-right,
bottom-left
*/
drawable.setCornerRadii(new float[] { r0, r0, r1, r1,
r2, r2, r3, r3 });
}
@Override protected void onDraw(Canvas canvas) {
mDrawable.setBounds(mRect);
float r = 35;
canvas.save();
canvas.translate(10, 10);
mDrawable.setGradientType(GradientDrawable.LINEAR_GRADIENT);
setCornerRadius(mDrawable, r, r, 0, 0);
mDrawable.draw(canvas);
canvas.restore();
canvas.translate(0, mRect.height() + 10);
canvas.save();
canvas.translate(10, 10);
mDrawable.setGradientType(GradientDrawable.RADIAL_GRADIENT);
setCornerRadius(mDrawable, 0, 0, r, r);
mDrawable.draw(canvas);
canvas.restore();
canvas.translate(0, mRect.height() + 10);
canvas.save();
canvas.translate(10, 10);
mDrawable.setGradientType(GradientDrawable.SWEEP_GRADIENT);
setCornerRadius(mDrawable, 0, r, r, 0);
mDrawable.draw(canvas);
canvas.restore();
}
}
}
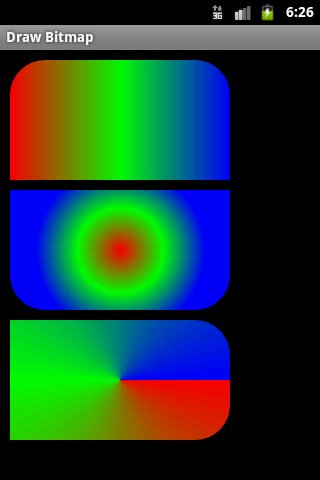
396LW NO topic_id
AD
Další témata ....(Topics)
Brand | Samsung |
Model (codename) | Galaxy Y (S5360) |
Cena, včetně DPH | 2400 / 06.2012 |
Display size (v palcích) | 3 |
Display-resolution | 240x320 |
Dotek-typ | kapacitní |
CPU typ | BCM21553 |
CPU MHz | 832 |
CPU core | |
L2 cache | ? |
RAM | 256 |
ROM | 512 |
GPU | VideoCore IV |
NenaMark2 Benchmark | 12 |
GPU-GLBenchmark | |
Baterie mAh | 1200 |
Foto MPx | 2 |
Autofocus | ne |
Video | |
Official Android ICS | Android OS v 2.3 |
CyanogenMod support | |
Dotek-prstů-max | |
Display-ppi | 133 |
Display-retina | 41% |
Network | čîtyřpásmový GSM 850/900/1800/1900 MHz , HSDPA 2100 MHz |
Connectivity | Bluetooth v2.0 plus EDR plus support A2DP, USB host |
Pozn. | CPU ARMv6, GPU 20MT/s OpenGL ES 2.0 |
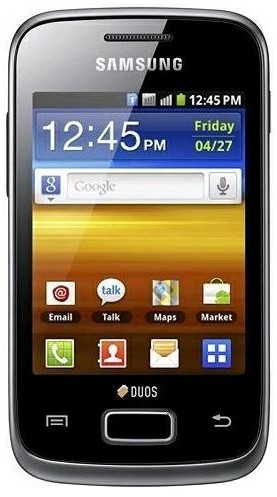
Canvas, drawCircle(), Paint, onDraw(), setStrokeWidth(), setStyle()
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
canvas.drawColor(Color.CYAN);
Paint p = new Paint();
// smooths
p.setAntiAlias(true);
p.setColor(Color.RED);
p.setStyle(Paint.Style.STROKE);
p.setStrokeWidth(4.5f);
// opacity
//p.setAlpha(0x80); //
canvas.drawCircle(50, 50, 30, p);
}
}
}
Try this:
- check names of drawables (file name must contain only abc...xyz 012...789 _ . in Resources folder ,
names have to start with lower case
MyImage.jpg == problem ,
names with space
my image.jpg == problem,
names with -
my-image.png == problem)
- check duplicate names with other extension ( my_image.jpg - my_image.png makes the problem)
- restart Android Studio
- if problem persist:
- check c:\Users\me\AndroidStudioProjects\myProject\myModule\build\intermediates\res\merged\debug\ drawable folder for corupted names or delete ALL drawable folders
- synk projekt, rebuild projekt
- if problem persist:
- restart Android Studio, wait for complete closure of the Android Studio!
- check names of drawables (file name must contain only abc...xyz 012...789 _ . in Resources folder ,
names have to start with lower case
MyImage.jpg == problem ,
names with space
my image.jpg == problem,
names with -
my-image.png == problem)
- check duplicate names with other extension ( my_image.jpg - my_image.png makes the problem)
- restart Android Studio
- if problem persist:
- check c:\Users\me\AndroidStudioProjects\myProject\myModule\build\intermediates\res\merged\debug\ drawable folder for corupted names or delete ALL drawable folders
- synk projekt, rebuild projekt
- if problem persist:
- restart Android Studio, wait for complete closure of the Android Studio!
Problemi is in e.getMessage() what can return null and Log.e (String tag, String msg) will throws an new exception !!!!
Problem and solution:
LogCat:
Or you can using this code:
Problem and solution:
try {
int [] i = {1};
int z = i[5];
} catch (ArrayIndexOutOfBoundsException e) {
String s = e.toString(); // s == java.lang.ArrayIndexOutOfBoundsException
// try to testing String s for null value
if(s != null)
Log.e("bla", s);
else
Log.e("bla", "My error text 1");
String s2 = e.getMessage(); // s2 == null !!!!!!!
// you need to testing String s2 for null value , or you get FATAL EXCEPTION: main
// and application will be crashed
String s2 = e.getMessage(); // s2 == null !!!!!!!
if(s2 != null)
Log.e("bla2", e.getMessage());
else
Log.e("bla2", "My error text 2");
// this is OK
e.printStackTrace();
}
LogCat:
E/bla(855): java.lang.ArrayIndexOutOfBoundsException
E/bla2(855): My error text 2
W/System.err(855): java.lang.ArrayIndexOutOfBoundsException
W/System.err(855): at cz.okhelp.motion._MotionActivity.onTouchEvent(_MotionActivity.java:54)
W/System.err(855): at android.app.Activity.dispatchTouchEvent(Activity.java:2099)
W/System.err(855): at com.android.internal.policy.impl.PhoneWindow$DecorView.dispatchTouchEvent(PhoneWindow.java:1675)
W/System.err(855): at android.view.ViewRoot.deliverPointerEvent(ViewRoot.java:2194)
W/System.err(855): at android.view.ViewRoot.handleMessage(ViewRoot.java:1878)
W/System.err(855): at android.os.Handler.dispatchMessage(Handler.java:99)
W/System.err(855): at android.os.Looper.loop(Looper.java:123)
W/System.err(855): at android.app.ActivityThread.main(ActivityThread.java:3683)
W/System.err(855): at java.lang.reflect.Method.invokeNative(Native Method)
W/System.err(855): at java.lang.reflect.Method.invoke(Method.java:507)
W/System.err(855): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:839)
W/System.err(855): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:597)
W/System.err(855): at dalvik.system.NativeStart.main(Native Method)
Or you can using this code:
try {
int [] i = {1};
int z = i[5];
} catch (Exception e) {
StringBuilder sb = new StringBuilder().append(e.getClass().getSimpleName());
if (e.getMessage() != null) {
sb.append("
");
sb.append(e.getMessage());
}
Log.e("err", sb.toString()); // E/err(336): ArrayIndexOutOfBoundsException
// this code write out all message
Log.e("myError", "methodName", e);
}
// E/myError(371): methodName
// E/myError(371): java.lang.ArrayIndexOutOfBoundsException
// E/myError(371): at cz.okhelp.motion._MotionActivity.onTouchEvent(_MotionActivity.java:54)
// E/myError(371): at android.app.Activity.dispatchTouchEvent(Activity.java:2099)
// E/myError(371): at com.android.internal.policy.impl.PhoneWindow$DecorView.dispatchTouchEvent(PhoneWindow.java:1675)
// E/myError(371): at android.view.ViewRoot.deliverPointerEvent(ViewRoot.java:2194)
// E/myError(371): at android.view.ViewRoot.handleMessage(ViewRoot.java:1878)
// E/myError(371): at android.os.Handler.dispatchMessage(Handler.java:99)
// E/myError(371): at android.os.Looper.loop(Looper.java:123)
// E/myError(371): at android.app.ActivityThread.main(ActivityThread.java:3683)
// E/myError(371): at java.lang.reflect.Method.invokeNative(Native Method)
// E/myError(371): at java.lang.reflect.Method.invoke(Method.java:507)
// E/myError(371): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:839)
// E/myError(371): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:597)
// E/myError(371): at dalvik.system.NativeStart.main(Native Method)
Why the app selects data from basic layout folder if smallest width is higher then the number in folder name?
Example 1
layout-sw600dp values-sw600dp (smallest width sw for data usage from this folder is 600dp density independent pixel!!!!!)
Device screen resolution is 1200 x 900 px (pixel) Wow, app to be select data from sw600dp folder! Realy?
DPI of device screen - dot per inch (pixel per inch) is 480 pixel it is wery important number!
In our case smallest dimension of screen must be at least 1800 real - physical pixels (1800 px / 3 ratio(dpi/160) = 600 dp (dip density independend pixels) to be used data from folders values-sw600dp and layout-sw600dp.
Example 2 see Example 1 abouve
Device: Nexus 7 (2012) selected from Android Studio tool layout editor
Resolution: 800x1280 px
DPI: tvdpi (approximately 213dpi)
Ratio: 1.33 (213 / 160)
Smallest width in px: 800
Convert px to dp: 601.5 (800 / 1.33)
Result:Smallest width is 601.5dp The App to be used data from folders values-sw600dp and layout-sw600dp.
Example 1
layout-sw600dp values-sw600dp (smallest width sw for data usage from this folder is 600dp density independent pixel!!!!!)
Device screen resolution is 1200 x 900 px (pixel) Wow, app to be select data from sw600dp folder! Realy?
DPI of device screen - dot per inch (pixel per inch) is 480 pixel it is wery important number!
- App selects smallest dimension of screen. In our case 900 px
Medium screen have 160 dpi (The density-independent pixel is equivalent to one physical pixel on a 160 dpi screen, which is the baseline density assumed by the system for a "medium" density screen.). - App calculate ratio 480 / 160 = 3 (The conversion of dp units to screen pixels: px = dp * (dpi / 160))
- App calculate smallest dimesnion of screen in dp 900 / 3 = 300 dip or dp (density independed pixel).
- App selects data from basic values and layout folder because sw600dp is greater than 300dp.
In our case smallest dimension of screen must be at least 1800 real - physical pixels (1800 px / 3 ratio(dpi/160) = 600 dp (dip density independend pixels) to be used data from folders values-sw600dp and layout-sw600dp.
Example 2 see Example 1 abouve
Device: Nexus 7 (2012) selected from Android Studio tool layout editor
Resolution: 800x1280 px
DPI: tvdpi (approximately 213dpi)
Ratio: 1.33 (213 / 160)
Smallest width in px: 800
Convert px to dp: 601.5 (800 / 1.33)
Result:Smallest width is 601.5dp The App to be used data from folders values-sw600dp and layout-sw600dp.
Editace: 2013-12-09 13:09:03
Počet článků v kategorii: 396
Url:bitmap-canvas-color-gradient-linear-radial-sweep-rounded-corner-android-example