How to create icon for Android apps
First cteate big icon 512x512 px formtat .PGN in graphics editor as Photoshop, Gimp, Piant.NET and save image.
If you create new project via Eclipse and choice your 512x512 image for ic_launcher.
Eclipse will make all icons from this image for new application very well.
If you create new project via Eclipse and choice your 512x512 image for ic_launcher.
Eclipse will make all icons from this image for new application very well.
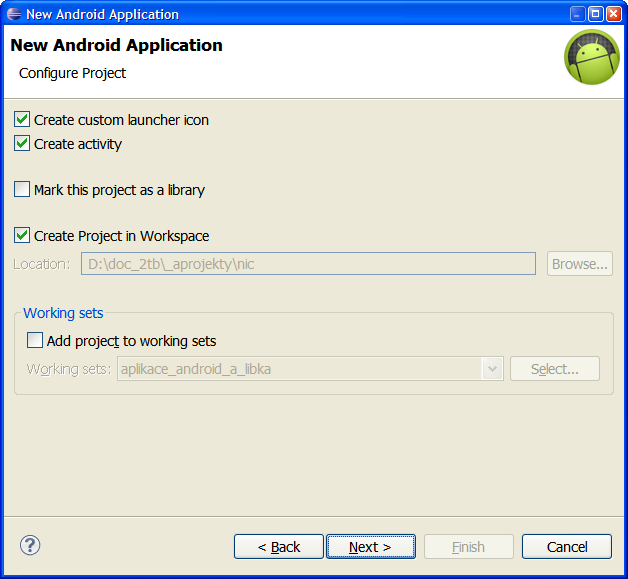
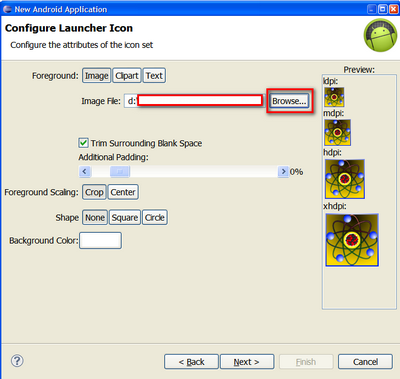
396LW NO topic_id
AD
Další témata ....(Topics)
6. Fragments Tutorial Summary – Czech language
Dil 6. shrnutí
V 1. dílu jsme se něco dozvěděli od XML souborech a typu procesoru pro správný běh Android Studia a emulátoru různých typů zařizení s Androidem.
- FrameLayout: můžeme měnit obsah kontejneru
- fragment s plnou cestou: nelze měnit obsah za jiný fragment
- pozor na šírku kontejnerů:
Složku layout-large lze přejmenovat na swXXXdp např. sw600dp a pak si zařízení bere automaticky resource z této složky, pokud nejmenší rozměr obrazovky je roven, či větší 600dp.
To lze využít k oklamání zařízení při testování. Pokud máte jen malý telefon a chcete na něm zkoušet dva panely, tak složku přejmenujte, po dobu testování, např. na sw300dp a pak i malé zařízení zobrazí oba panely. Po ukončení testování je třeba zase složku přejmenovat na původní nejmenší přípustnou šířku zařízení (w600dp), nebo nejmenší rozměr jedné ze stran obrazovky (sw600dp).
V 2. dílu jsme rozebrali MainActivity.java
Pozor na implementaci správné třídy, pokud si vytvoříte novou třídu se seznamem položek,
je třeba přejemnova HeadlinesFragment na NovyNazevTridyKdeMateListView
implements HeadlinesFragment.OnHeadlineSelectedListener
na
implements NovyNazevTridyKdeMateListView.OnHeadlineSelectedListener
V 3. dílu jsme se zabývali HeadlinesFragment.java
V 4. dílu jsme se podívali na ArticleFragment.java
Fragment používa onCreateView() místo onCreate()
V 5. dílu jsme probrali uložiště řetězců Ipsum.java
Ipsum je veřejná třída, která obsahuje
dvě pole řetězců - stringů.
Pole Headlines slouží jako uložiště pro názvy, které
budou načteny do ListView - seznamu v HeadlinesFragment.java
Pole Articles je v našem případě zásobárnou článků, které
budou načteny dle pozice položky ListView předané z HeadlinesFragment
zoětbě do MainActivity a
odtud do ArticleFragment.java, jako parametr metody
articleFrag.updateArticleView(position);
nebo jako argument Bundle
Bundle args = new Bundle();
args.putInt(ArticleFragment.ARG_POSITION, position);
Stringy - ukládat do souboru java je ošemetné (problémy s kódováním, vyhledávání výrazů atd.)
U většího množství článků pak nepřehledné.
Navíc, uživatel nemůže tento text editovat.
K ukládaní většího množství dat, k jejich vyhledávání
a editaci je lépe používat databáze.
Dil 6. shrnutí
V 1. dílu jsme se něco dozvěděli od XML souborech a typu procesoru pro správný běh Android Studia a emulátoru různých typů zařizení s Androidem.
- FrameLayout: můžeme měnit obsah kontejneru
- fragment s plnou cestou: nelze měnit obsah za jiný fragment
- pozor na šírku kontejnerů:
android:layout_width="0dp" - musí být 0dp, jinak se např. některý panel nezobrazí, nebo neuvídíte vůbec nic.
android:layout_weight="1" u prvního panelu fragmentu
android:layout_weight="2" u panelu druhého fragmentu
Složku layout-large lze přejmenovat na swXXXdp např. sw600dp a pak si zařízení bere automaticky resource z této složky, pokud nejmenší rozměr obrazovky je roven, či větší 600dp.
To lze využít k oklamání zařízení při testování. Pokud máte jen malý telefon a chcete na něm zkoušet dva panely, tak složku přejmenujte, po dobu testování, např. na sw300dp a pak i malé zařízení zobrazí oba panely. Po ukončení testování je třeba zase složku přejmenovat na původní nejmenší přípustnou šířku zařízení (w600dp), nebo nejmenší rozměr jedné ze stran obrazovky (sw600dp).
V 2. dílu jsme rozebrali MainActivity.java
Pozor na implementaci správné třídy, pokud si vytvoříte novou třídu se seznamem položek,
je třeba přejemnova HeadlinesFragment na NovyNazevTridyKdeMateListView
implements HeadlinesFragment.OnHeadlineSelectedListener
na
implements NovyNazevTridyKdeMateListView.OnHeadlineSelectedListener
Fragmenty lze měnit ve FrameLayout kontejneru
// MALÉ OBRAZOVKY
// jen JEDEN-PANEL se zobrazuje - vybrán soubor news_articles.xml v layout složce
// musíme vyměnit fragemnty !!!!
// Vytvoříme fragment a doplníme argumenty - hodnoty, poslané např. z HeadlinesFragment.java
// jedná se nám především o pozici položky, na kterou bylo kliknuto v ListView
// tato pozice bude určující pro výběr obsahu pro ArticleFragment
ArticleFragment newFragment = new ArticleFragment();
Bundle args = new Bundle();
args.putInt(ArticleFragment.ARG_POSITION, position);
newFragment.setArguments(args);
FragmentTransaction transaction = getSupportFragmentManager().beginTransaction();
// Zde vyměníme původní fragment HeadlinesFragment novým fragmentem s obsahem článku atd.
// Můžeme vyměnit fragment za jiný, protože id fragment_container v layout/news_articles.xml je tagu FrameLayout
transaction.replace(R.id.fragment_container, newFragment);
// addToBackStack umožní uživateli vrátit se zpět na seznam položek v HeadlinesFragment.java
transaction.addToBackStack(null);
// celou transakci předáme ke schválení :)
transaction.commit();
V 3. dílu jsme se zabývali HeadlinesFragment.java
// zajistí zpětné odesílání zprav - zde zachytí kliknutí uživatele na položku
// v ListView - seznamu položek
OnHeadlineSelectedListener mCallback;
// pro odposlouchávání zpráv v ListView a odesílání do MainActivity.java
public interface OnHeadlineSelectedListener {
/**
funkce je volána onArticleSelected z HeadlinesFragment když uživatel
klikne na item v ListView - položku seznamu -
Tělo funkce je v MainActivity!!! Tam proběhnou potřebné úkony.
Například výměna fragmentů atd. */
public void onArticleSelected(int position);
}
/........
// novější verze ListView má více možností
int layout = Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB ?
android.R.layout.simple_list_item_activated_1 : android.R.layout.simple_list_item_1;
/........
// podbarvení vybrané položky v ListView zlepší přehlednost
// u dvou panelového zobrazeni
/** kliknuto na položku ListView */
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
// long id se bude hodit například při obsluze sql.databází
// Upozorní - předá zprávu MainActivity.java, že uživatel
// klikl na položku a odešle i pozici v seznamu
mCallback.onArticleSelected(position);
// getListView().setItemChecked(position, true);
// má zajistit podbarvení
// - zvýraznění vybrané položky,
// ale mi to nefungovalo, tak jsem ještě znovu přidal,
// které je už v onStart() a už to funguje jak má
// Možná nějaká záludnost v mém telefonu :(
getListView().setChoiceMode(ListView.CHOICE_MODE_SINGLE);
getListView().setItemChecked(position, true);
}
@Override
public void onStart() {
super.onStart();
// Pokud se bude zobrazovat dual-panel
// (pro tablety, větší obrazovky)
// například je dobré
// když bude zvýrazněna vybraná položka setChoiceMode(ListView.CHOICE_MODE_SINGLE);
// Toto provádíme v onStart(), kdy máme přístup k listview
if (getFragmentManager().findFragmentById(R.id.article_fragment) != null) {
getListView().setChoiceMode(ListView.CHOICE_MODE_SINGLE);
}
}
V 4. dílu jsme se podívali na ArticleFragment.java
Fragment používa onCreateView() místo onCreate()
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Když je activity znovu vytvořena (např. při rotaci zařízení),
// obnoví, v našem případě, text článku, jehož pozice
// byla uložena pomocí
// public void onSaveInstanceState(Bundle outState) viz níže
// důležité zejména pro dual-panel (dva panely vedle sebe)
if (savedInstanceState != null) {
mCurrentPosition = savedInstanceState.getInt(ARG_POSITION);
}
// umístíme, aktivujeme příslušný layout
// zde je zajímavé, že layout můžete měnit.
// Např. při kliknutí na pložku 1 v HeadlinesFragment
// zde můžete ochytit pozici a dle toho zvolit
// příslušný layout, který chcete zobrazit ve fragmentu
// ALE pak si musíte pohlídat ID prvků, které bude ten JINÝ
// layout obsahovat
return inflater.inflate(R.layout.article_view, container, false);
}
// na rozdíl od Activity se ve Fragment používá k
// získání id ne jen findViewById()
// ALE getActivity().findViewById()
TextView article = (TextView) getActivity().findViewById(R.id.article);
@Override
public void onStart() {
super.onStart();
// Při startu fragmentu, zkontrolujte, zda existují nějaké argumenty
// předané do fragmentu.
// OnStart() je právě to správné místo, kde to udělat,
// protože layout s jednotlivými elementy byl již
// naloděn - aktivován, a můžeme bezpečně použít metody,
// které potřebují, aby jednotlivá ID elementů layoutu byla již
// aktivní, použitelná a nevracela NULL, což by mělo za následek
// pád aplikace
Bundle args = getArguments();
if (args != null) {
// vypsaní obsahu článku pomocí předaného argumentu (pozice) z HeadlinesFragment.java
updateArticleView(args.getInt(ARG_POSITION));
} else if (mCurrentPosition != -1) {
// vypsání článku dle pozice uložené např. při rotaci zařízení
// mCurrentPosition je definována (odchycena) v onCreateView
updateArticleView(mCurrentPosition);
}
}
V 5. dílu jsme probrali uložiště řetězců Ipsum.java
Ipsum je veřejná třída, která obsahuje
dvě pole řetězců - stringů.
Pole Headlines slouží jako uložiště pro názvy, které
budou načteny do ListView - seznamu v HeadlinesFragment.java
Pole Articles je v našem případě zásobárnou článků, které
budou načteny dle pozice položky ListView předané z HeadlinesFragment
zoětbě do MainActivity a
odtud do ArticleFragment.java, jako parametr metody
articleFrag.updateArticleView(position);
nebo jako argument Bundle
Bundle args = new Bundle();
args.putInt(ArticleFragment.ARG_POSITION, position);
Stringy - ukládat do souboru java je ošemetné (problémy s kódováním, vyhledávání výrazů atd.)
U většího množství článků pak nepřehledné.
Navíc, uživatel nemůže tento text editovat.
K ukládaní většího množství dat, k jejich vyhledávání
a editaci je lépe používat databáze.
String sFileContent = readFile("myfile.txt",StandardCharsets.UTF_8);
static String readFile(String path, Charset encoding)
throws IOException
{
byte[] encoded = Files.readAllBytes(Paths.get(path));
return encoding.decode(ByteBuffer.wrap(encoded)).toString();
}
// write file
String sOut = "text blah hello world etc.";
writeToFile(sOut"someName.txt");
static void writeToFile(String sB,String name) {
String folder = ("c:\\folder\");
File f = new File(folder+ name);
BufferedWriter writer = null;
writer = new BufferedWriter( new OutputStreamWriter(
new FileOutputStream( folder+name),"UTF-8"));
writer.write( sB);
if ( writer != null)
writer.close( );
}
Check
- is sett debug mode on device?
- enabled debugging over USB?
- is device right connected?
- have you downloaded and instaled drivers for your device on PC?
- For Windows //developer.android.com/sdk/win-usb.html
- try disconnect and connect USB cable
- try do restart Android Studio
drawText(), setColor(), setTextSize(), setTextAlign()
public class ApokusActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
paint.setColor(Color.YELLOW);
paint.setTextSize(60);
//paint.setTextAlign(Paint.Align.CENTER);
canvas.drawText("Hello world!", 0, 50, paint);
}
}
}
1.) Open DDMS via Menu Tools > Android > Android Device Monitor
2.) Select Device
3.) In DDMS click Menu Window > Show View > File Exlporer
4.) Device > Storage folder
5.) Emulator > data > data folder
2.) Select Device
3.) In DDMS click Menu Window > Show View > File Exlporer
4.) Device > Storage folder
5.) Emulator > data > data folder
Editace: 2013-12-09 13:01:14
Počet článků v kategorii: 396
Url:how-to-create-icon-for-android-apps