How get bitmap dimension without loading Android example
Bitmap Width, Height without memory allocation:
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeResource(getResources(), R.id.myimage, options);
// now opts.outWidth and opts.outHeight are the dimension of the
// bitmap, even though Bitmap is null
int imageHeight = options.outHeight;
int imageWidth = options.outWidth;
String imageType = options.outMimeType;
396LW NO topic_id
AD
Další témata ....(Topics)
How to quickly change all icon set in .apk project with Eclipse:
Right click on project in folder tree
Select New - Ohter
In open wizard dialog select Android Icon Set
Set Icon set name:
Open some large icon for your project for example 512x512 px and wizard will make all set of icons from one largest icon:
Wizard will create all set of icons from this one largest icon.
Click on finish button:
Right click on project in folder tree
Select New - Ohter

In open wizard dialog select Android Icon Set
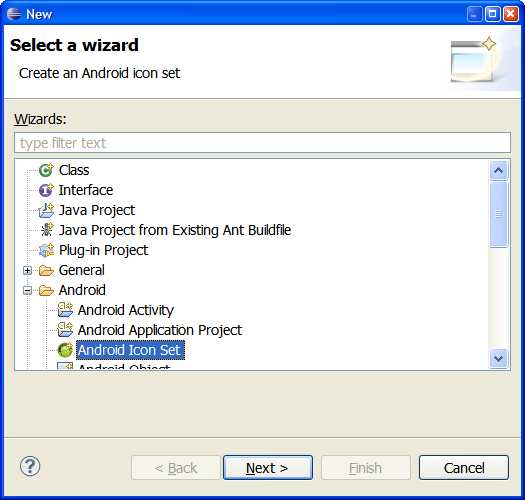
Set Icon set name:
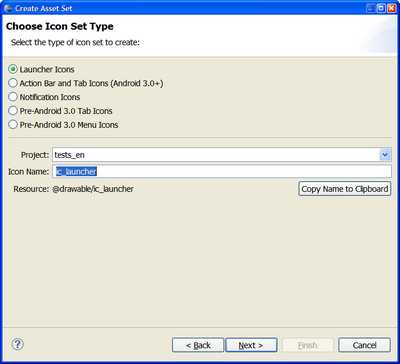
Open some large icon for your project for example 512x512 px and wizard will make all set of icons from one largest icon:
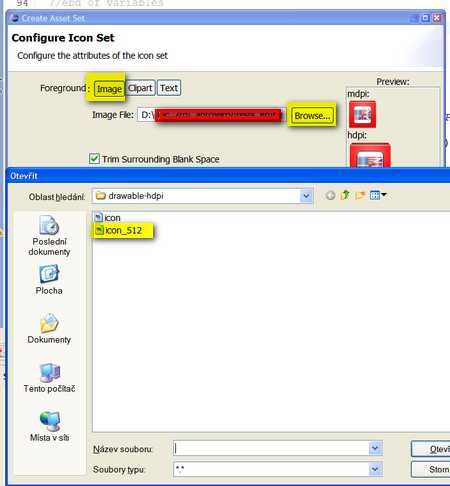
Wizard will create all set of icons from this one largest icon.
Click on finish button:
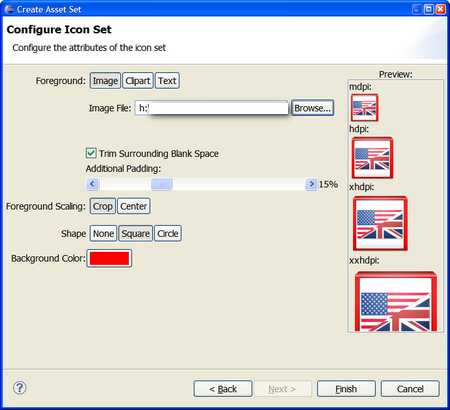
Convert number int to string Android Java example source code.
int n = 0;
try {
n = Integer.parseInt("21"));
} catch(NumberFormatException e) {
System.out.println("Could not parse " + e);
}
// int to string
String s = String.valueOf(24);
public class ApokusActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
canvas.drawColor(Color.YELLOW);
paint.setFilterBitmap(true);
Bitmap bitmapOrg = BitmapFactory.decodeResource(getResources(),R.drawable.flower_blue);
int targetWidth = bitmapOrg.getWidth() * 2;
int targetHeight = bitmapOrg.getHeight() * 2;
Bitmap bmp = Bitmap.createBitmap(targetWidth, targetHeight,Bitmap.Config.ARGB_8888);
RectF rectf = new RectF(0, 0, targetWidth, targetHeight);
Canvas c = new Canvas(bmp);
Path path = new Path();
path.addRect(rectf, Path.Direction.CW);
c.clipPath(path);
c.drawBitmap( bitmapOrg, new Rect(0, 0, bitmapOrg.getWidth(), bitmapOrg.getHeight()),
new Rect(0, 0, targetWidth, targetHeight), paint);
Matrix matrix = new Matrix();
matrix.postScale(1f, 1f);
Bitmap resizedBitmap = Bitmap.createBitmap(bmp, 0, 0, targetWidth, targetHeight, matrix, true);
int h = bitmapOrg.getHeight();
canvas.drawBitmap(bitmapOrg, 10,10, paint);
canvas.drawBitmap(resizedBitmap, 10,10 + h + 10, paint);
}
}
}
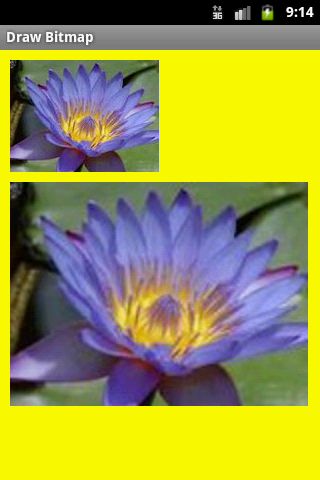
Example have error code:
//developer.android.com/training/basics/fragments/creating.html
Try to change ArticleFragment.java
//developer.android.com/training/basics/fragments/creating.html
Try to change ArticleFragment.java
/*
* Copyright (C) 2012 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* //www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.example.android.fragments;
import android.support.v4.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
public class ArticleFragment extends Fragment {
final static String ARG_POSITION = "position";
int mCurrentPosition = -1;
TextView articleText;
@Override
// public View onCreateView(LayoutInflater inflater, ViewGroup container,
// Bundle savedInstanceState) {
//
// // If activity recreated (such as from screen rotate), restore
// // the previous article selection set by onSaveInstanceState().
// // This is primarily necessary when in the two-pane layout.
// if (savedInstanceState != null) {
// mCurrentPosition = savedInstanceState.getInt(ARG_POSITION);
// }
//
// // Inflate the layout for this fragment
// return inflater.inflate(R.layout.article_view, container, false);
// }
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// If activity recreated (such as from screen rotate), restore
// the previous article selection set by onSaveInstanceState().
// This is primarily necessary when in the two-pane layout.
if (savedInstanceState != null) {
mCurrentPosition = savedInstanceState.getInt(ARG_POSITION);
}
// Inflate the layout for this fragment
View rootView = inflater.inflate(R.layout.article_view, container, false);
articleText = (TextView) rootView.findViewById(R.id.article);
return rootView;
}
@Override
public void onStart() {
super.onStart();
// During startup, check if there are arguments passed to the fragment.
// onStart is a good place to do this because the layout has already been
// applied to the fragment at this point so we can safely call the method
// below that sets the article text.
Bundle args = getArguments();
if (args != null) {
// Set article based on argument passed in
updateArticleView(args.getInt(ARG_POSITION));
} else if (mCurrentPosition != -1) {
// Set article based on saved instance state defined during onCreateView
updateArticleView(mCurrentPosition);
}
}
public void updateArticleView(int position) {
//TextView article = (TextView) getActivity().findViewById(R.id.article); //Error: article=null.
if (articleText != null)
articleText.setText(Ipsum.Articles[position]);
mCurrentPosition = position;
}
/* ERROR public void updateArticleView(int position) {
TextView article = (TextView) getActivity().findViewById(R.id.article);
article.setText(Ipsum.Articles[position]);
mCurrentPosition = position;
}*/
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
// Save the current article selection in case we need to recreate the fragment
outState.putInt(ARG_POSITION, mCurrentPosition);
}
}
Go to Eclipse menu:
Window -> Preferences -> Java -> Code Style -> Formatter
Press NEW profile or EDIT if have you some profile.
Setup your settings.
Save settings.
Window -> Preferences -> Java -> Code Style -> Formatter
Press NEW profile or EDIT if have you some profile.
Setup your settings.
Save settings.

Editace: 2013-12-09 13:00:05
Počet článků v kategorii: 396
Url:how-get-bitmap-dimension-without-loading-android-example