Rotate Canvas with Bitmap Android example
drawPath, canvas.rotate, lineTo basic Android example for your testing.
![]() |
|
![]() |
|
// //www.apache.org/licenses/LICENSE-2.0
// The Android Open Source Project
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static class SampleView extends View {
private Paint mPaint = new Paint();
private Path mPath = new Path();
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
// Construct a wedge-shaped path
mPath.moveTo(0, -60);
mPath.lineTo(-20, 80);
mPath.lineTo(0, 60);
mPath.lineTo(20, 80);
mPath.close();
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = mPaint;
canvas.drawColor(Color.WHITE);
paint.setAntiAlias(true);
paint.setColor(Color.RED);
paint.setStyle(Paint.Style.FILL);
Bitmap bitmapOrg = BitmapFactory.decodeResource(getResources(),
R.drawable.flower_blue);
canvas.drawBitmap(bitmapOrg, 10, 10, paint);
int w = canvas.getWidth();
int h = canvas.getHeight();
int cx = w / 2;
int cy = h / 2;
canvas.translate(cx, cy);
// uncomment next line
//canvas.rotate(90.0f);
canvas.drawPath(mPath, mPaint);
}
}
}
396LW NO topic_id
AD
Další témata ....(Topics)
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new SampleView(this));
}
private static Bitmap codec(Bitmap src, Bitmap.CompressFormat format,
int quality) {
ByteArrayOutputStream os = new ByteArrayOutputStream();
src.compress(format, quality, os);
byte[] array = os.toByteArray();
return BitmapFactory.decodeByteArray(array, 0, array.length);
}
private static class SampleView extends View {
// CONSTRUCTOR
public SampleView(Context context) {
super(context);
setFocusable(true);
}
@Override
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
canvas.drawColor(Color.YELLOW);
Bitmap bmp = BitmapFactory.decodeResource(getResources(), R.drawable.flower_blue);
Bitmap bPNGcompress = codec(bmp, Bitmap.CompressFormat.PNG, 0);
int h = bmp.getHeight();
canvas.drawBitmap(bmp, 10,10, paint);
canvas.drawBitmap(bPNGcompress, 10,10 + h + 10, paint);
}
}
}
Check
- is sett debug mode on device?
- enabled debugging over USB?
- is device right connected?
- have you downloaded and instaled drivers for your device on PC?
- For Windows //developer.android.com/sdk/win-usb.html
- try disconnect and connect USB cable
- try do restart Android Studio
Error for example:
webcoreglue(2075): The real object has been deleted
Solution:
If this error message shows if orientation the screen is changed
try insert into AndroidManifest.xml this code:
android:configChanges="keyboard|keyboardHidden|orientation"
webcoreglue(2075): The real object has been deleted
Solution:
If this error message shows if orientation the screen is changed
try insert into AndroidManifest.xml this code:
android:configChanges="keyboard|keyboardHidden|orientation"
<activity
android:label="@string/app_name"
android:name=".MyActivity"
android:screenOrientation="portrait"
android:configChanges="keyboard|keyboardHidden|orientation"
>
Eclipse Navigation Shortcuts.
Keyboard_shortcuts_(3.0).pdf
Ctrl+1 Quick fix problem
Ctrl+O Quick Outline
Ctrl+Shift+G Find references in workspace
Ctrl+Shift+U Find all references in file
Ctrl+Shift+T Find Java Typ
[caption id="attachment_1214" align="alignleft" width="150" caption="eclipse-ctrl-shift-t"]
[/caption]
Ctrl+Shift+R Find Resource
Ctrl+E Open Editor Drop-Down
Ctrl+Space Content Assist
Ctrl+Shift+Space Context Information
F3 Open Declaration of variable
F4 Open Type Hierarchy
Ctrl+H Open Search Dialog
Alt+Shift+R Rename - Refactoring
Alt+Shift+M Extract Method
Alt+Shift+L Extract Local Variable
Alt Shift Up Expand selection to enclosing element
Alt Shift Right Expand selection to next element
Alt Shift Left Expand selection to previous element
Alt Shift Down Restore previous selection
Breakpoints Shift+Alt+Q B
Cheat Sheets Shift+Alt+Q H
Console Shift+Alt+Q C
Java Declaration Shift+Alt+Q D
Java Package Explorer Shift+Alt+Q P
Java Type Hierarchy Shift+Alt+Q T
Javadoc Shift+Alt+Q J
Search Shift+Alt+Q S
Show View (View: Outline)Shift+Alt+Q O
Show View (View: Problems)Shift+Alt+Q X
Synchronize Shift+Alt+Q Y
Variables Shift+Alt+Q V

Keyboard_shortcuts_(3.0).pdf
Ctrl+1 Quick fix problem
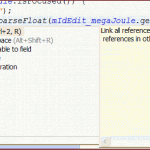
Ctrl+O Quick Outline

Ctrl+Shift+G Find references in workspace
Ctrl+Shift+U Find all references in file

Ctrl+Shift+T Find Java Typ
[caption id="attachment_1214" align="alignleft" width="150" caption="eclipse-ctrl-shift-t"]
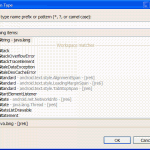
Ctrl+Shift+R Find Resource

Ctrl+E Open Editor Drop-Down
Ctrl+Space Content Assist

Ctrl+Shift+Space Context Information
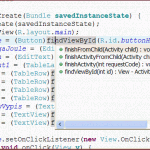
F3 Open Declaration of variable
F4 Open Type Hierarchy
Ctrl+H Open Search Dialog
Alt+Shift+R Rename - Refactoring
Alt+Shift+M Extract Method
Alt+Shift+L Extract Local Variable
Alt Shift Up Expand selection to enclosing element
Alt Shift Right Expand selection to next element
Alt Shift Left Expand selection to previous element
Alt Shift Down Restore previous selection
Breakpoints Shift+Alt+Q B
Cheat Sheets Shift+Alt+Q H
Console Shift+Alt+Q C
Java Declaration Shift+Alt+Q D
Java Package Explorer Shift+Alt+Q P
Java Type Hierarchy Shift+Alt+Q T
Javadoc Shift+Alt+Q J
Search Shift+Alt+Q S
Show View (View: Outline)Shift+Alt+Q O
Show View (View: Problems)Shift+Alt+Q X
Synchronize Shift+Alt+Q Y
Variables Shift+Alt+Q V

do while Java example
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "Hello", "people", "hello", "world!" };
int i = 0;
// first cycle is always executed
do {
System.out.println("Loop: " + i);
System.out.println(arrayOfString[i]);
i++;
}while ( i == -1 );
}
}
/*
Loop: 0
Hello
*/
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = { "Hello", "people", "hello", "world!" };
int i = 0;
do {
System.out.println("Loop: " + i);
System.out.println(arrayOfString[i]);
i++;
}while ( i < arrayOfString.length );
}
}
/*
Loop: 0
Hello
Loop: 1
people
Loop: 2
hello
Loop: 3
world!
*/
Editace: 2013-12-09 13:08:53
Počet článků v kategorii: 396
Url:rotate-canvas-with-bitmap-android-example