break statement in Java Android example
break statement in Java Android basic example
MainClass.java
MainClass.java
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = {"nothing", "Hello", "people"
, "bye-bye", "hello", "world!", "end" };
for (int i = 0; i < arrayOfString.length; i++) {
System.out.println(arrayOfString[i]);
if(i > 2)
break; // end of loop
}
}
}
/*
nothing
Hello
people
bye-bye
*/
396LW NO topic_id
AD
Další témata ....(Topics)
The Android WebView changing the height according to text size.
If you need to change the height to a minimum try this source:
If you need to change the height to a minimum try this source:
//public void loadDataWithBaseURL (String baseUrl, String data, String mimeType, String encoding, String historyUrl)
WebView _webView; // findById(.....
String entryContent = "";
_webView.loadDataWithBaseURL(
"blabol",// javascript i styly
entryContent, null,
"utf-8", null
);
InputStream, getResources(),openRawResource()
java.io.InputStream is;
is = context.getResources().openRawResource(R.drawable.my_image);
Drawable mDrawable = context.getResources().getDrawable(R.drawable.button);
mDrawable.setBounds(150, 20, 300, 100);
Drawable[] mDrawables;
int[] resIDs = new int[] {
R.drawable.btn_ok,
R.drawable.btn_storno,
R.drawable.btn_help
};
mDrawables = new Drawable[resIDs.length];
Drawable prev = mDrawable;
for (int i = 0; i < resIDs.length; i++) {
mDrawables[i] = context.getResources().getDrawable(resIDs[i]);
mDrawables[i].setDither(true);
addToTheRight(mDrawables[i], prev);
prev = mDrawables[i];
}
Code look up at the Api Demos
android_fragments arguments attributes - three fragments on screen
android_fragments_alert_dialog
android_fragments_context_menu
android_fragments_custom_animation
android_fragments_dialog
android_fragments_dialog_or_activity
fragment_hide_show
fragment_layout
fragments_list_array
fragment_menu
android_fragments_get_result_from_fragment and tabs in two rows
android_fragments_recive_result
android_fragments_stack
fragment_tabs
android_fragments arguments attributes - three fragments on screen
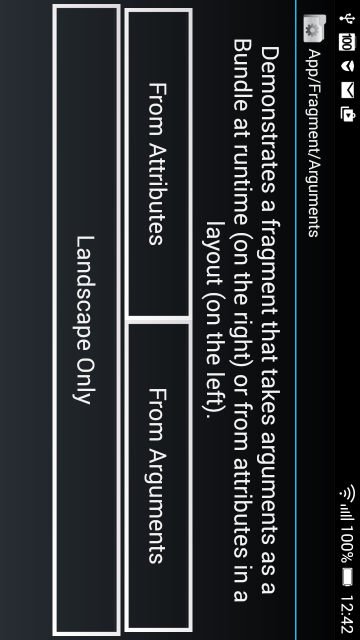
android_fragments_alert_dialog
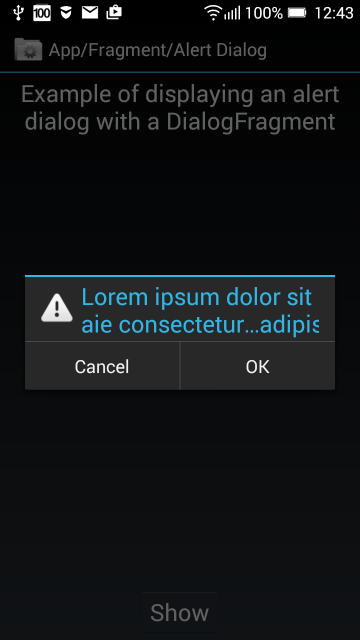
android_fragments_context_menu
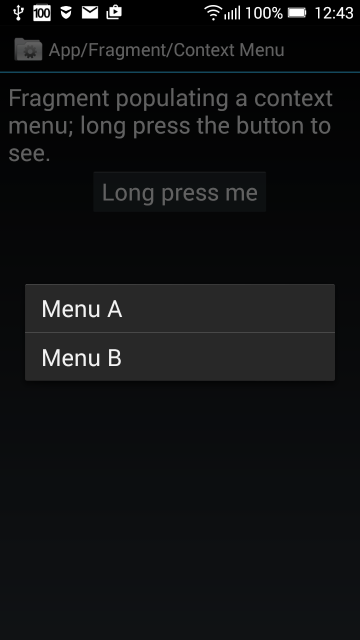
android_fragments_custom_animation
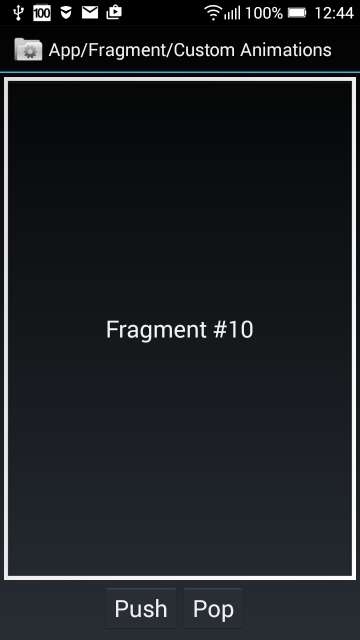
android_fragments_dialog

android_fragments_dialog_or_activity
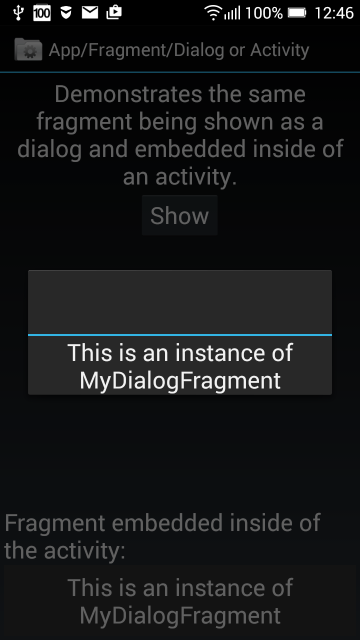
fragment_hide_show

fragment_layout

fragments_list_array
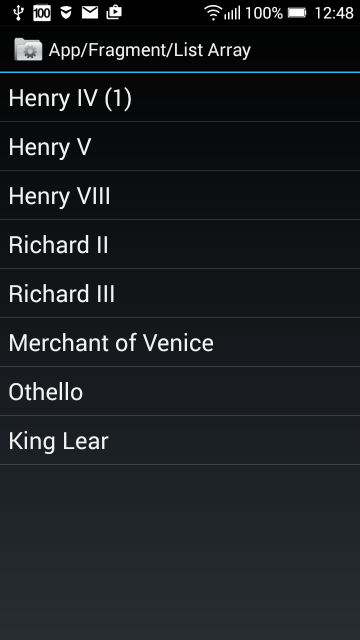
fragment_menu

android_fragments_get_result_from_fragment and tabs in two rows
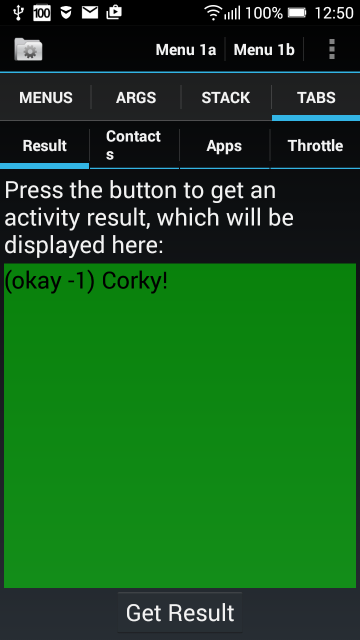
android_fragments_recive_result
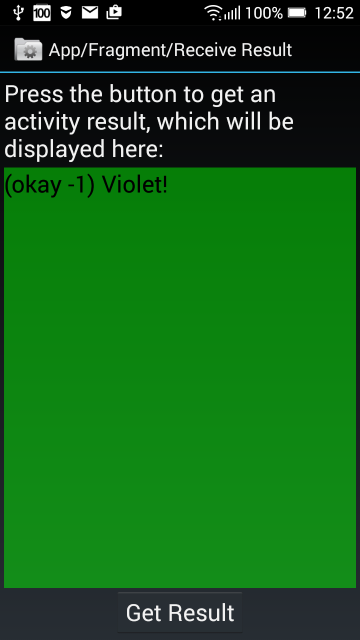
android_fragments_stack

fragment_tabs

Try this:
- check names of drawables (file name must contain only abc...xyz 012...789 _ . in Resources folder ,
names have to start with lower case
MyImage.jpg == problem ,
names with space
my image.jpg == problem,
names with -
my-image.png == problem)
- check duplicate names with other extension ( my_image.jpg - my_image.png makes the problem)
- restart Android Studio
- if problem persist:
- check c:\Users\me\AndroidStudioProjects\myProject\myModule\build\intermediates\res\merged\debug\ drawable folder for corupted names or delete ALL drawable folders
- synk projekt, rebuild projekt
- if problem persist:
- restart Android Studio, wait for complete closure of the Android Studio!
- check names of drawables (file name must contain only abc...xyz 012...789 _ . in Resources folder ,
names have to start with lower case
MyImage.jpg == problem ,
names with space
my image.jpg == problem,
names with -
my-image.png == problem)
- check duplicate names with other extension ( my_image.jpg - my_image.png makes the problem)
- restart Android Studio
- if problem persist:
- check c:\Users\me\AndroidStudioProjects\myProject\myModule\build\intermediates\res\merged\debug\ drawable folder for corupted names or delete ALL drawable folders
- synk projekt, rebuild projekt
- if problem persist:
- restart Android Studio, wait for complete closure of the Android Studio!
How add pair of strings to Hashtable, how get pair key value from Hashtable, how split string, basic Java Android example.
MainClass.java
MainClass.java
import java.util.Enumeration;
import java.util.Hashtable;
public class MainClass {
public static void main(String[] arg) {
// english;germany dictionary
String[] arrayOfString = { "one;eine", "two;zwei", "three;drei" };
Hashtable<String, String> hashTable = new Hashtable<String, String>();
for(String s: arrayOfString){
String[] array = s.split(";");
String sKey ="", sValue="";
if(array.length > 1){
sKey = array[0]; sValue = array[1];
hashTable.put(sKey, sValue);
}
}
Enumeration<String> enumer = hashTable.keys();
while (enumer.hasMoreElements()) {
String keyFromTable = (String) enumer.nextElement();
// get Returns the value to which the specified key is mapped,
// or null if this map contains no mapping for the key
System.out.println(keyFromTable + " = " + hashTable.get(keyFromTable));
}
}
}
/*
two = zwei
one = eine
three = drei
*/
Editace: 2011-10-04 10:49:22
Počet článků v kategorii: 396
Url:break-statement-in-java-android-example