Linkify text link url in TextView text Android example
Example from SDK C:\Program Files\Android\android-sdk-windows\samples\android-10\ApiDemos\src\com\example\android\apis\text\Link.java
Source: //developer.android.com/resources/browser.html?tag=sample
License: //www.apache.org/licenses/LICENSE-2.0
1.) Automatically linkifies using android:autoLink="all"
2.) Link text by setMovementMethod
3.) Link as html code using Html.fromHtml()
4.) Link string by SpannableString
Source: //developer.android.com/resources/browser.html?tag=sample
License: //www.apache.org/licenses/LICENSE-2.0
1.) Automatically linkifies using android:autoLink="all"
// res/values/strings.xml
<string name="link_text_auto"><b>text1:</b> This is some text. In
this text are some things that are actionable. For instance,
you can click on //www.google.com and it will launch the
web browser. You can click on google.com too. And, if you
click on (415) 555-1212 it should dial the phone.
</string>
// main.xml
<!-- text1 automatically linkifies things like URLs and phone numbers. -->
<TextView xmlns:android="//schemas.android.com/apk/res/android"
android:id="@+id/text1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:autoLink="all"
android:text="@string/link_text_auto"
/>
2.) Link text by setMovementMethod
// MainActivity.java onCreate
/*Be warned that if you want a TextView with a key listener or movement method not to be focusable, or if you want a TextView without a key listener or movement method to be focusable, you must call setFocusable(boolean) again after calling this to get the focusability back the way you want it. */
TextView t2 = (TextView) findViewById(R.id.text2);
t2.setMovementMethod(LinkMovementMethod.getInstance());
// main.xml
<!-- text2 uses a string resource containing explicit <a> tags to
specify links. -->
<TextView xmlns:android="//schemas.android.com/apk/res/android"
android:id="@+id/text2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="@string/link_text_manual"
/>
//strings.xml
<string name="link_text_manual"><b>text2:</b> This is some other
text, with a <a href="//www.google.com">link</a> specified
via an <a> tag. Use a "tel:" URL
to <a href="tel:4155551212">dial a phone number</a>.
</string>
3.) Link as html code using Html.fromHtml()
// MainActivity.java onCreate
TextView t3 = (TextView) findViewById(R.id.text3);
t3.setText(
Html.fromHtml(
"<b>text3:</b> Text with a " +
"<a href="//www.google.com">link</a> " +
"created in the Java source code using HTML."));
t3.setMovementMethod(LinkMovementMethod.getInstance());
4.) Link string by SpannableString
SpannableString ss = new SpannableString(
"text4: Click here to dial the phone.");
ss.setSpan(new StyleSpan(Typeface.BOLD), 0, 6,
Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
ss.setSpan(new URLSpan("tel:4155551212"), 13, 17,
Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
TextView t4 = (TextView) findViewById(R.id.text4);
t4.setText(ss);
t4.setMovementMethod(LinkMovementMethod.getInstance());
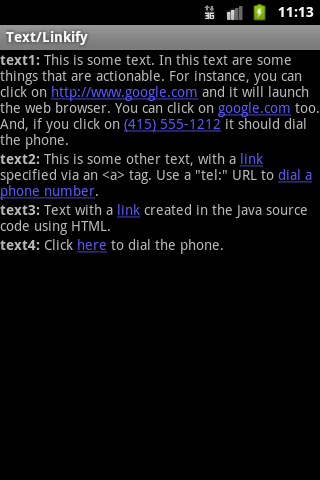
396LW NO topic_id
AD
Další témata ....(Topics)
List LinkedList Collections add sort find search Item, get searched item to string in Java example.
MainClass.java
MainClass.java
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
public class MainClass {
public static void main(String[] arg) {
String[] arrayOfString = {"nothing", "Hello", "people"
, "bye-bye", "hello", "world!", "End" };
List<String> arrayList = new LinkedList<String>();
for(String s: arrayOfString)
arrayList.add(s);
Collections.sort(arrayList);
// foreach
for (String str: arrayList)
System.out.println(str);
Object objMin = Collections.min(arrayList);
System.out.println("Min is: " + objMin);
Object objMax = Collections.max(arrayList);
System.out.println("Max is: " + objMax);
int index = Collections.binarySearch(arrayList, "people");
System.out.println("Index of people is: " + index);
// print word on index 5
System.out.println("Index 5 is: " + arrayList.get(5));
String sItem = arrayList.get(5); // get item from index 5 to string
}
}
/*
End
Hello
bye-bye
hello
nothing
people
world!
Min is: End
Max is: world!
Index of people is: 5
Index 5 is: people
*/
If Android emulator freezes at startup, try create new virtual device with smaller memory and cpu usage, or select older version of Android, or buy new pc with higher performance.
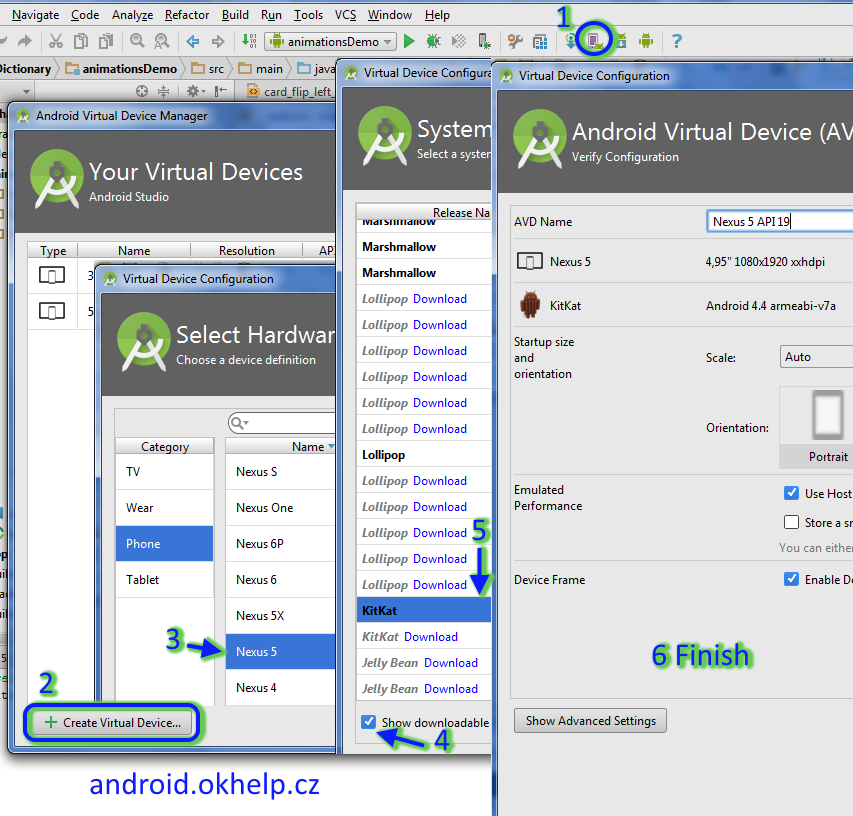
- open AVD MANAGER
- press Create Virtual Device
- select Device with small memory usage (e.g. 480x800 of resolution) - Next
- check - Show downloadable ....
- Download - lower version of system Android, select, press - Next
- check data, Show Advanced Settings, check Use host... and press Finish
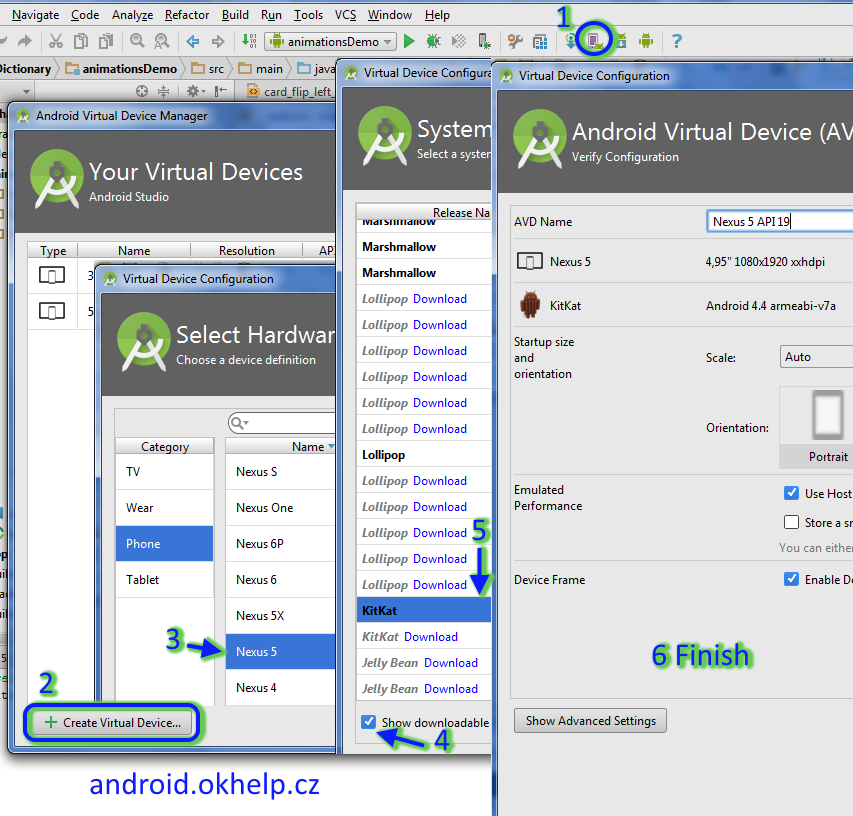
public class Main extends Activity {
private TextView mTextView;
private Activity mAct;
private Intent mIntent;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_layout);
mTextView = findViewById(R.id.mTextView);
mAct = getActivity();
mIntent = getIntent();
}
}
to:
public class Main extends Fragment{
private TextView mTextView;
private FragmentActivity mFrgAct;
private Intent mIntent;
private LinearLayout mLinearLayout;
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View root = inflater.inflate(R.layout.fragment_main, null);
return root;
}
public void onViewCreated(View view, Bundle savedInstanceState) {
// you can add listener of elements here
/*Button mButton = (Button) view.findViewById(R.id.button);
mButton.setOnClickListener(this); */
mTextView = view.findViewById(R.id.mTextView);
mLinearLayout = (LinearLayout)view;
}
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
mFrgAct = getActivity();
mIntent = mFrgAct.getIntent(); // Intent intent = new Intent(getActivity().getIntent());
}
}
Check if in drawable folder have two picture with same name and delete one.
For example:
Check the module build folder in Android Studio for two files with same names.
For example:
image_1.jpg
image_1.png
Check the module build folder in Android Studio for two files with same names.
C:\Users\myFolder\AndroidStudioProjects\Project\yourModule\build\intermediates\res\merged\debug\drawable\
In your project AndroidManifest.xml you can set permission of Android application example source code.
For example if you want allow your application connection to INTERNET you have to permit this in AndroidManifest.xml.
WebViev show error: Website Not Available you have to permit INTERNET
For example if you want allow your application connection to INTERNET you have to permit this in AndroidManifest.xml.
WebViev show error: Website Not Available you have to permit INTERNET
<uses-permission
android:name="android.permission.INTERNET" />
<uses-permission
android:name="android.permission.GET_ACCOUNTS" />
<uses-permission
android:name="android.permission.USE_CREDENTIALS" />
<uses-permission
android:name="android.permission.MANAGE_ACCOUNTS" />
<uses-permission
android:name="android.permission.AUTHENTICATE_ACCOUNTS" />
<uses-permission
android:name="android.permission.WRITE_SETTINGS" />
<uses-permission
android:name="android.permission.WRITE_SECURE_SETTINGS" />
<uses-permission
android:name="android.permission.READ_CONTACTS" />
<uses-permission
android:name="android.permission.WRITE_CONTACTS" />
<uses-permission
android:name="android.permission.READ_SYNC_STATS" />
<uses-permission
android:name="android.permission.READ_SYNC_SETTINGS" />
<uses-permission
android:name="android.permission.WRITE_SYNC_SETTINGS" />
Editace: 2011-11-25 12:32:18
Počet článků v kategorii: 396
Url:linkify-text-link-url-in-textview-text-android-example